This C# program illustrates left-shift operations, which are bitwise operations used to shift the bits of an integer to the left. Left-shifting a number by a certain number of positions effectively multiplies it by 2 raised to the power of the shift amount. Left shifts can be useful in various scenarios, including optimizing mathematical operations, setting or clearing specific bits, and more.
Problem Statement
You are given an integer num
and an integer shiftCount
. Your task is to perform a left shift operation on num
by shiftCount
positions and print the result in binary and decimal form.
C# Program to Demonstrate Left-Shift Operations
using System; class Program { static void Main() { // Demonstrating left shift operations int num = 10; // Binary: 00001010 // Left shifting the number by 2 positions int result1 = num << 2; // Binary: 00101000, Decimal: 40 Console.WriteLine($"Left shift by 2 positions: {result1}"); // Left shifting the number by 3 positions int result2 = num << 3; // Binary: 01010000, Decimal: 80 Console.WriteLine($"Left shift by 3 positions: {result2}"); // Left shifting the number by 1 position using a loop int num2 = 5; // Binary: 00000101 for (int i = 0; i < 4; i++) { num2 = num2 << 1; // Left shift by 1 position in each iteration Console.WriteLine($"Left shift iteration {i + 1}: {num2}"); } } }
How it Works
- User Input: The program starts by prompting the user to input two integer values:
num
andshiftCount
. These values are read from the console using theConsole.ReadLine()
method and converted to integers usingint.Parse()
. - Display Original Number: The program displays the original number
num
in both binary and decimal form. To displaynum
in binary form, it uses theConvert.ToString()
method with base 2 to convert the integer into a binary string. It also displaysnum
as-is in decimal form. - Left Shift Operation: The program performs a left shift operation on
num
byshiftCount
positions using the<<
operator. This operation shifts the binary representation ofnum
to the left, effectively multiplyingnum
by 2^shiftCount
. The result is stored in the variableresult
. - Display Shifted Number: The program displays the shifted number
result
in both binary and decimal form, similar to how it displayed the original number. Again, it usesConvert.ToString()
to convert the integer result into a binary string. - Display Shift Count: Finally, the program prints the number of positions shifted, which is simply the value of
shiftCount
. - Validation: Although not shown in the code snippet, in a real-world application, you should consider adding input validation to ensure that
shiftCount
is non-negative and that the user inputs are within the appropriate range. - End of Program: The program execution ends, and the results are displayed to the user.
Input/ Output
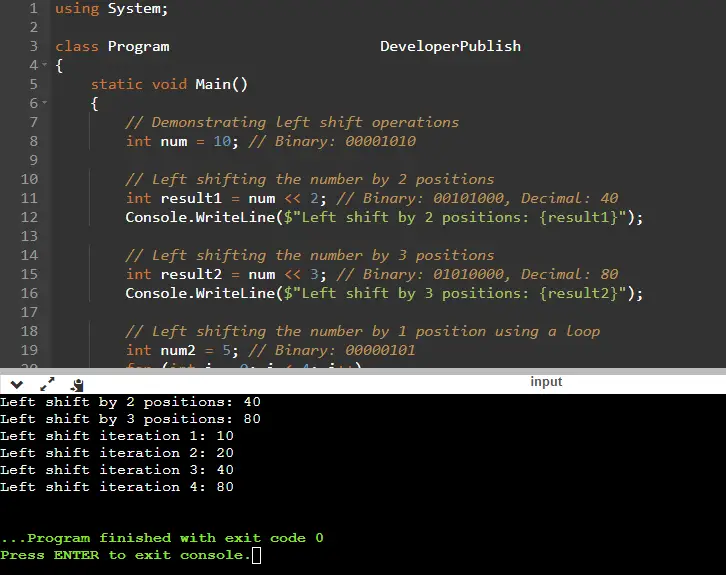