This C program demonstrates how to delete a specific line from a file. It takes the filename and the line number as input from the user and deletes the corresponding line from the file.
Problem statement
Given a file and a line number, write a C program to delete the specified line from the file.
C Program to Delete a Specific Line from File
#include <stdio.h> #include <stdlib.h> void deleteLineFromFile(const char* filename, int lineNum) { // Function to delete a specific line from a file // ... int main() { const char* filename = "example.txt"; int lineNum; printf("Enter the line number to delete: "); scanf("%d", &lineNum); deleteLineFromFile(filename, lineNum); return 0; }
How it works?
- The program defines a function
deleteLineFromFile
that takes the filename and line number as parameters. - It opens the input file in read mode and a temporary file in write mode.
- The program reads each line from the input file and writes all lines except the line to be deleted into the temporary file.
- After reading all lines, it closes both files and deletes the original file.
- Finally, it renames the temporary file to the original file name, effectively replacing the original file with the modified version.
- In the
main
function, the program prompts the user to enter the line number to delete. - It calls the
deleteLineFromFile
function with the provided filename and line number.
Input/output
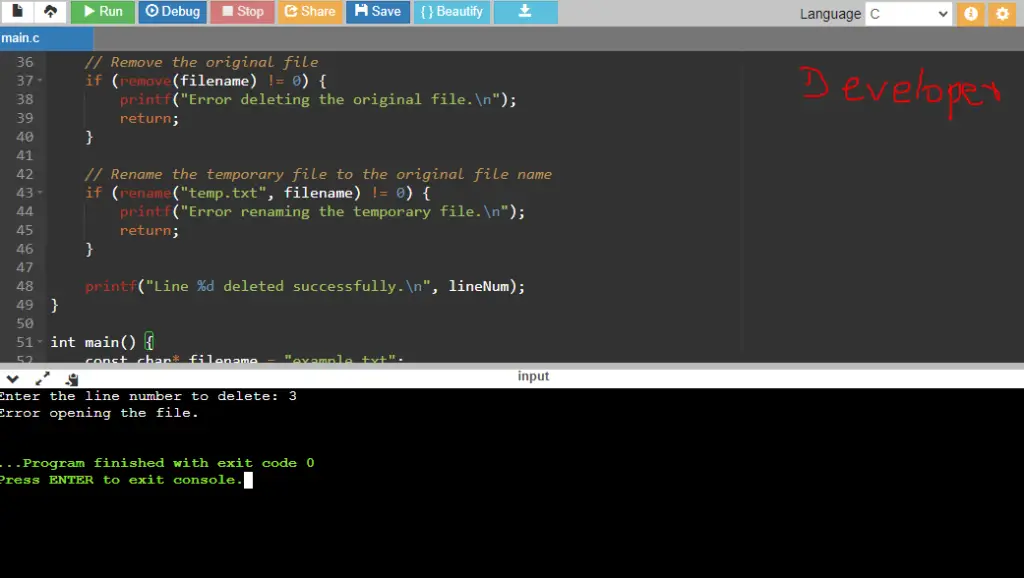