This C# program demonstrates the creation of a simple stopwatch using the Stopwatch
class from the System.Diagnostics
namespace.
Stopwatch is a timekeeping device used to measure the elapsed time between a start point and a stop point. In software development, creating a stopwatch typically involves designing and implementing a program or feature that can accurately measure and display elapsed time.
Problem Statement
Design and implement a stopwatch application that allows users to accurately measure and display elapsed time.
C# Program to Create a Stop Watch
using System; using System.Diagnostics; using System.Threading; class Program { static void Main() { Console.WriteLine("Press Enter to start the stopwatch..."); Console.ReadLine(); Stopwatch stopwatch = new Stopwatch(); stopwatch.Start(); while (true) { Console.Clear(); Console.WriteLine("Stopwatch: " + stopwatch.Elapsed.ToString("hh\\:mm\\:ss\\.fff")); Console.WriteLine("Press Enter to stop the stopwatch..."); if (Console.KeyAvailable && Console.ReadKey(true).Key == ConsoleKey.Enter) { stopwatch.Stop(); break; } Thread.Sleep(10); } Console.WriteLine("Stopwatch stopped. Press Enter to exit."); Console.ReadLine(); } }
How it Works
- Starting the Stopwatch:
- When you start a stopwatch, it records the current time as the starting point (usually in hours, minutes, seconds, and milliseconds).
- It typically displays “00:00:00.000” (or similar) as the initial time.
- Measuring Elapsed Time:
- As time progresses, the stopwatch continuously calculates the elapsed time by subtracting the starting time from the current time.
- It updates the display to show the elapsed time in real-time, with the format “hh:mm:ss.fff” (hours:minutes:seconds.milliseconds).
- Stopping the Stopwatch:
- When you stop the stopwatch, it records the current time as the ending point.
- It ceases to update the display with real-time values.
- Calculating Total Elapsed Time:
- The total elapsed time is calculated by subtracting the starting time from the ending time.
- This provides an accurate measure of the time that passed while the stopwatch was running.
- Resetting the Stopwatch:
- You can reset the stopwatch to zero, clearing both the starting and ending times and resetting the display to “00:00:00.000.”
- Recording Lap Times (Optional):
- Some stopwatches offer the feature to record lap times or split times.
- When you press a “lap” or “split” button, the current elapsed time is recorded and displayed separately from the main timer.
- This allows you to track intermediate times while the stopwatch continues running.
- User Interface:
- Stopwatch applications often provide a user-friendly interface with buttons or commands for starting, stopping, resetting, and recording laps.
- They may also include additional features like saving recorded times or exporting data.
- Accuracy:
- Stopwatch functionality relies on high-precision timekeeping mechanisms provided by the underlying hardware or software libraries.
- The accuracy of a stopwatch is crucial for applications that require precise time measurements.
- Display and Formatting:
- The stopwatch display typically formats the time in hours, minutes, seconds, and milliseconds.
- Formatting may include leading zeros (e.g., “05:03:12.045”) for consistency and readability.
- Error Handling:
- Stopwatch applications may include error handling to manage exceptional cases, such as invalid user inputs or unexpected behaviors.
Input Output
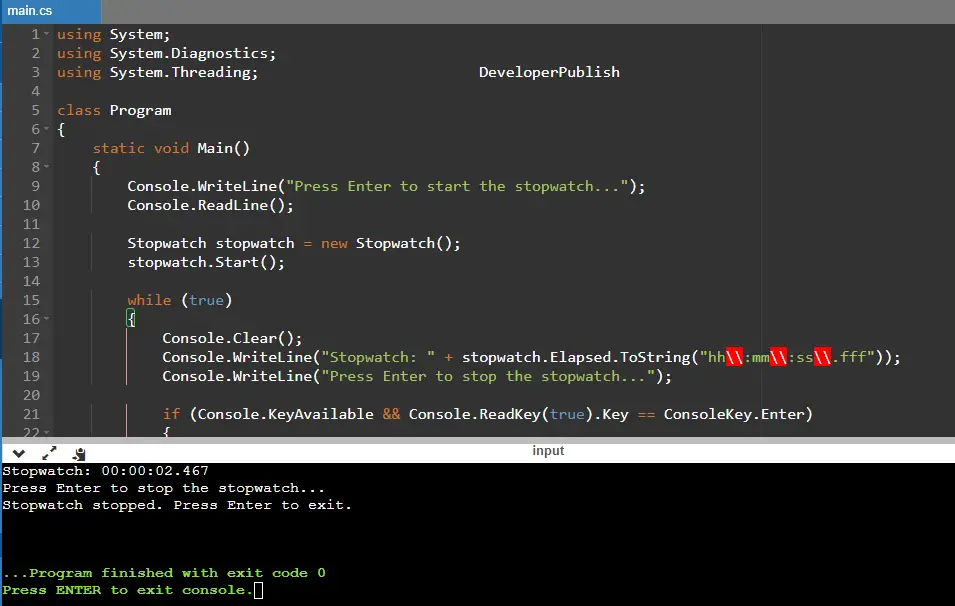