This C program takes the name of an input file as user input and converts its content to lowercase. The converted content is then saved in a new file called ‘output.txt’.
Problem Statement
Write a C program that reads the content of a file, converts it to lowercase, and saves the modified content in another file.
C Program to Convert the Content of File to Lowercase
#include <stdio.h> #include <ctype.h> #define MAX_SIZE 1000 int main() { FILE *inputFile, *outputFile; char fileName[100], ch; printf("Enter the name of the input file: "); scanf("%s", fileName); // Open the input file in read mode inputFile = fopen(fileName, "r"); if (inputFile == NULL) { printf("Cannot open file '%s'\n", fileName); return 1; } // Open the output file in write mode outputFile = fopen("output.txt", "w"); if (outputFile == NULL) { printf("Cannot create output file\n"); return 1; } // Read characters from input file and convert to lowercase while ((ch = fgetc(inputFile)) != EOF) { fputc(tolower(ch), outputFile); } printf("Content converted to lowercase and saved to 'output.txt'\n"); // Close the files fclose(inputFile); fclose(outputFile); return 0; }
How it works
- The program starts by asking the user to enter the name of the input file.
- It then attempts to open the input file in read mode using
fopen()
. If the file cannot be opened, an error message is displayed, and the program terminates. - Next, it creates an output file called ‘output.txt’ using
fopen()
in write mode. If the output file cannot be created, an error message is displayed, and the program terminates. - The program reads each character from the input file using
fgetc()
in a loop until it reaches the end of the file (EOF). - Inside the loop, each character is converted to lowercase using
tolower()
function, and the lowercase character is written to the output file usingfputc()
. - After the loop finishes, the program displays a success message and closes both the input and output files using
fclose()
. - Finally, the program terminates with a return value of 0.
Input / Output
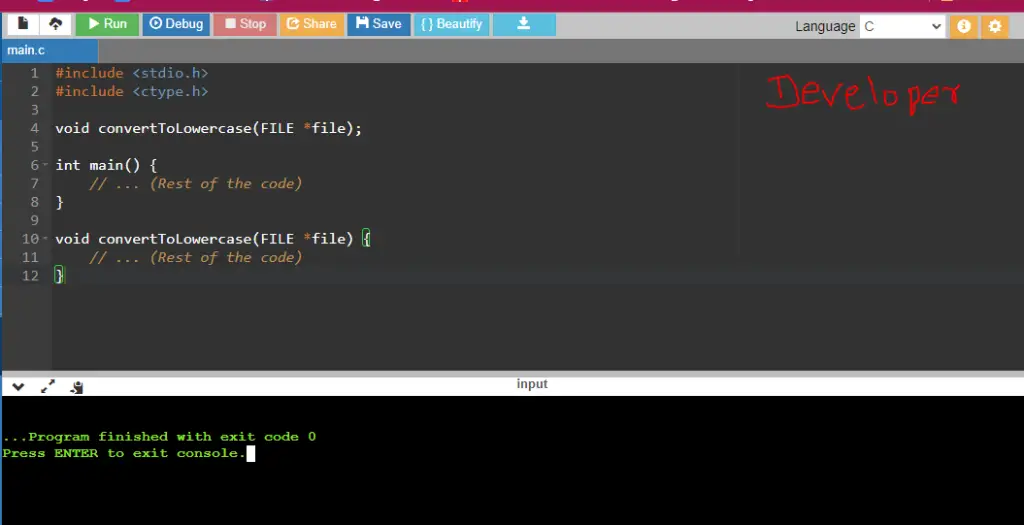