This C program converts a decimal number to its binary representation using recursion. It takes a decimal number as input and prints its binary equivalent.
Problem statement
Given a decimal number, we need to convert it to its binary representation using recursion.
C Program to Convert Decimal to Binary using Recursion
#include <stdio.h> void decimalToBinary(int num) { if (num > 0) { decimalToBinary(num / 2); printf("%d", num % 2); } } int main() { int decimalNum; printf("Enter a decimal number: "); scanf("%d", &decimalNum); printf("Binary representation: "); decimalToBinary(decimalNum); return 0; }
How it works
- The program prompts the user to enter a decimal number.
- The decimal number is stored in the variable
decimalNum
. - The
decimalToBinary
function is called withdecimalNum
as the argument. - Inside the
decimalToBinary
function:- If the number is greater than 0, it calls itself with the quotient
num / 2
. - It then prints the remainder
num % 2
, which gives the binary digit (0 or 1) at that position.
- If the number is greater than 0, it calls itself with the quotient
- After all the recursive calls, the binary representation is printed in reverse order.
- The
main
function prints the binary representation obtained by thedecimalToBinary
function. - The program terminates.
Input/Output
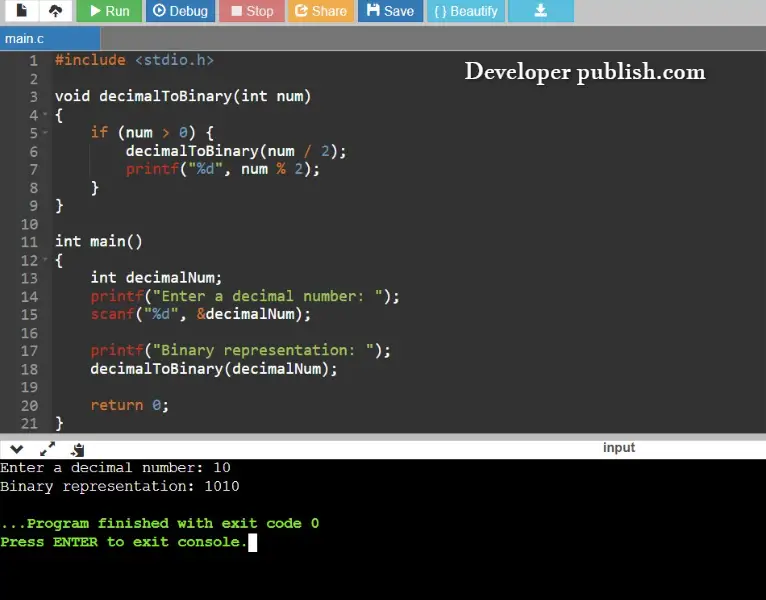