In this example, we’ll explore a simple C# program to determine if a given number is even or odd, showcasing the language’s ability to handle basic logic.
Problem Statement
Write a C# program to check whether a given integer is even or odd. The program should take an input number and determine if it’s divisible by 2. If it is, the number is even; otherwise, it’s odd.
C# Program to Check Whether a Given Number is Even or Odd
using System; class Program { static void Main() { Console.Write("Enter a number: "); int number = Convert.ToInt32(Console.ReadLine()); if (IsEven(number)) { Console.WriteLine(number + " is even."); } else { Console.WriteLine(number + " is odd."); } } static bool IsEven(int num) { return num % 2 == 0; } }
Input / Output
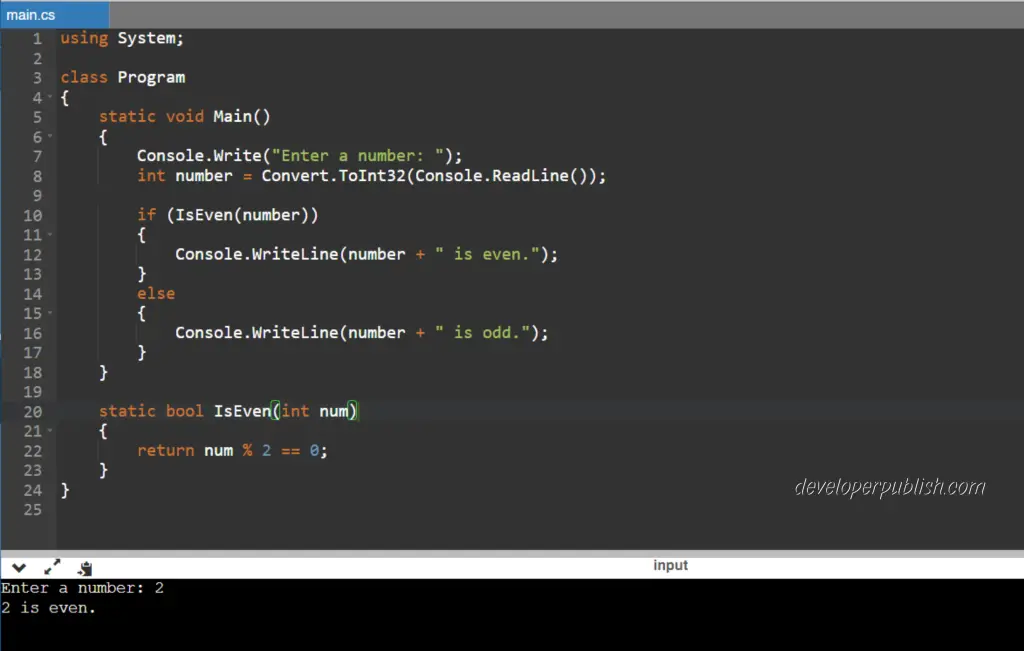