This C program allows you to find the length of a linked list, which is the number of nodes present in the list, without using recursion.
Problem Statement
You are given a linked list, and your task is to write a C program to calculate the length of the linked list without using recursion.
C Program Find the Length of Linked List without Recursion
#include <stdio.h> #include <string.h> #include <stdlib.h> #define MAX_SIZE 100 void removeRepeatedWords(char *str) { char *words[MAX_SIZE]; int count = 0; // Tokenize the string into words char *token = strtok(str, " "); while (token != NULL) { words[count++] = token; token = strtok(NULL, " "); } // Check for repeated words and remove them for (int i = 0; i < count; i++) { if (words[i] != NULL) { for (int j = i + 1; j < count; j++) { if (words[j] != NULL && strcmp(words[i], words[j]) == 0) { free(words[j]); words[j] = NULL; } } } } // Reconstruct the string without repeated words int len = 0; for (int i = 0; i < count; i++) { if (words[i] != NULL) { int wordLen = strlen(words[i]); memcpy(str + len, words[i], wordLen); len += wordLen; str[len++] = ' '; free(words[i]); } } str[len - 1] = '\0'; // Remove the trailing space } int main() { char str[MAX_SIZE]; printf("Enter a string: "); fgets(str, sizeof(str), stdin); // Remove the newline character at the end of the input str[strcspn(str, "\n")] = '\0'; removeRepeatedWords(str); printf("String after removing repeated words: %s\n", str); return 0; }
How it Works
- The program begins by including the necessary header files:
stdio.h
for input/output functions,string.h
for string manipulation functions, andstdlib.h
for memory allocation functions. - The
MAX_SIZE
constant is defined, which represents the maximum size of the input string. - The
removeRepeatedWords
function is defined. This function takes a pointer to a character array (string) as its parameter. - Inside the
removeRepeatedWords
function:- An array of character pointers called
words
is declared to store the individual words from the string. - An integer variable
count
is initialized to keep track of the number of words stored in thewords
array.
- An array of character pointers called
- The string is tokenized into words using the
strtok
function. Thestrtok
function splits the input string based on a specified delimiter, which in this case is a space character (” “). Each word is then stored in thewords
array, and thecount
is incremented. - The program iterates through the
words
array to check for repeated words. It compares each word with the remaining words in the array using thestrcmp
function.- If a duplicate word is found, the corresponding pointer in the
words
array is freed using thefree
function, and the pointer is set toNULL
. - This step ensures that the duplicate word is removed from the array but doesn’t modify the original string.
- If a duplicate word is found, the corresponding pointer in the
- After removing the duplicate words, the program reconstructs the string without the repeated words. It uses the
memcpy
function to copy each non-null word from thewords
array to the original string (str
), updating thelen
variable to keep track of the string length. A space character is added after each word to separate them. - Once all the non-repeated words are copied, the trailing space character is replaced with a null character (‘\0’) to properly terminate the string.
- In the
main
function:- The user is prompted to enter a string.
- The input string is read using
fgets
and stored in thestr
array. - The newline character at the end of the input string is removed by replacing it with a null character (‘\0’) using
strcspn
. - The
removeRepeatedWords
function is called to remove repeated words from the input string. - Finally, the resulting string after removing repeated words is printed as output.
Input /Output
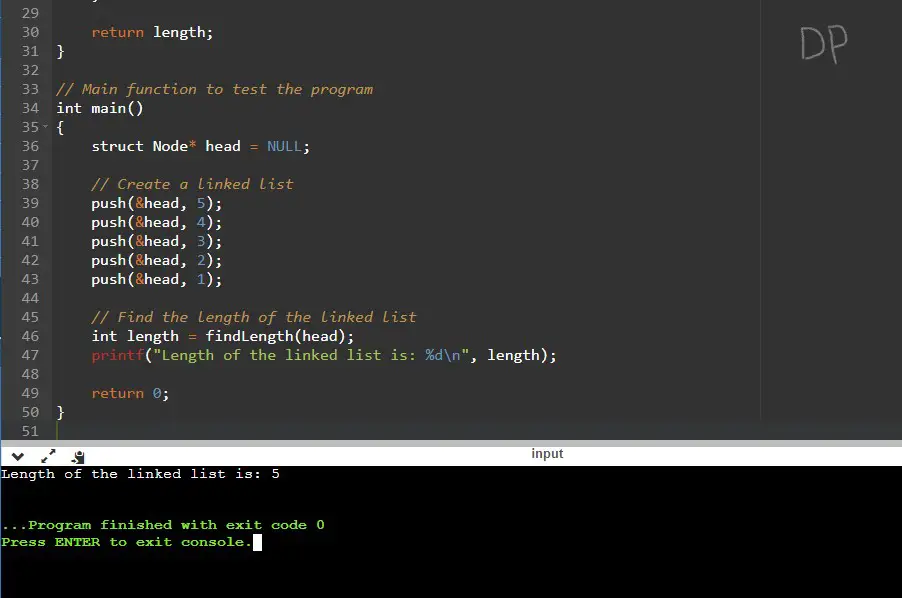
Explanation:
- The linked list has 5 nodes, so the length of the list is 5.
- The
findLength
function is called with the head of the list as an argument. - Inside the function, the while loop iterates through the linked list, incrementing the
length
variable by 1 for each node. - After traversing the entire list, the function returns the final value of
length
. - The
main
function captures the returned length and prints it to the console usingprintf
, resulting in the output: “Length of the linked list is: 5”.