Assume that you need to retrieve some device information from Windows Phone. You can get them from the classes “DeviceExtendedProperties” and “DeviceStatus” defined in the Microsoft.Phone.Info namespace.
Note that i am using the Real Windows Phone Device to retreive the information .
How to Retreive the Device Information from Windows Phone ?
The DeviceExtendedProperties allows the App to retreive the info about the device on which it is running .
With the DeviceExtendedProperties , it is possible to get the device info from the following properties
- DeviceName
- DeviceUniqueId
- DeviceManufacturer
- ApplicationCurrentMemoryUsage
- ApplicationPeakMemoryUsage
- DeviceName
- DeviceUniqueId
- DeviceFirmwareVersion
- DeviceHardwareVersion
- DeviceTotalMemory
We can use the TryGetValue method of the DeviceExtendedProperties class to get the info as below .
public void GetDeviceName() { string output = string.Empty; object MyDeviceName; if (DeviceExtendedProperties.TryGetValue("DeviceName", out MyDeviceName)) { output = MyDeviceName.ToString(); } MessageBox.Show(MyDeviceName.ToString()); }
The DeviceExtendedProperties was the one that was used in the WP7 but with the WP7.1 SDK , it is suggested to use the DeviceStatus instead .
According to MSDN Documentation .
“DeviceExtendedProperties is deprecated. Use DeviceStatus instead.”
In Windows Phone Mango , the DeviceStatus static class is used to get the device info .
Apart from the properties like DeviceName , DeviceTotalMemory , DeviceFirmwareVersion , ApplicationCurrentMemoryUsage , ApplicationPeakMemoryUsage , DeviceHardwareVersion , DeviceManufacturer , the devicestatus also provides the provides following properties
- IsKeyboardDeployed
- IsKeyboardPresent
- PowerSource .
You can also get the Windows Phone Operating System Version number from Environment.OSVersion .
public MainPage() { InitializeComponent(); GetDeviceInfo(); } public void GetDeviceInfo() { long ApplicationMemoryUsage = DeviceStatus.ApplicationCurrentMemoryUsage; long PeakMemoryUsage = DeviceStatus.ApplicationPeakMemoryUsage; string FirmwareVersion = DeviceStatus.DeviceFirmwareVersion; string HardwareVersion = DeviceStatus.DeviceHardwareVersion; string Manufacturer = DeviceStatus.DeviceManufacturer; string DeviceName = DeviceStatus.DeviceName; long TotalMemory = DeviceStatus.DeviceTotalMemory; string OSVersion = Environment.OSVersion.Version.ToString(); ; PowerSource powerSource = DeviceStatus.PowerSource; AddToList("Memory Usage :" + ApplicationMemoryUsage); AddToList("Peak Memory Usage :" + PeakMemoryUsage); AddToList("Firmware Version :" + FirmwareVersion); AddToList("Hardware Version :" + HardwareVersion); AddToList("Manufacturer :" + Manufacturer); AddToList("Total Memory :" + TotalMemory); AddToList("Power Source:" + powerSource.ToString()); AddToList("Operating System: Windows Phone " + OSVersion.ToString()); } public void AddToList(string Property) { lstboxDeviceInfo.Items.Add(Property); }
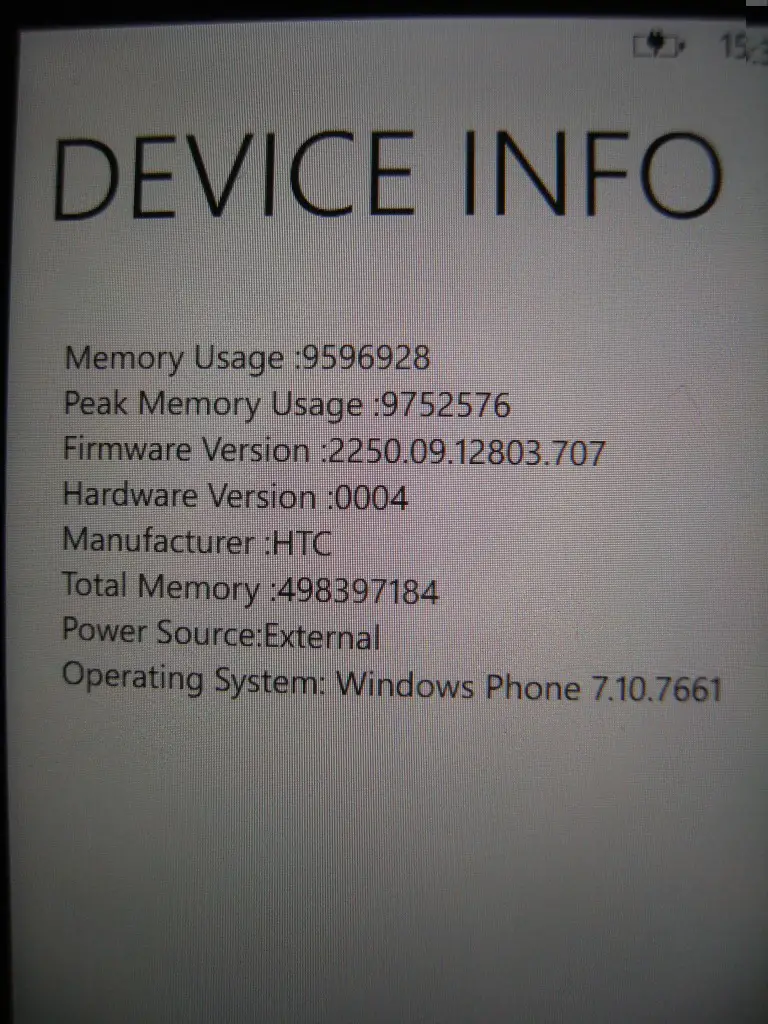
Note that the device OS Version we get is 7.1 but the Phone has 7.5 defined in the settings .
Reference :
1 Comment
thanks