This Python program checks if a given date is valid or not. It verifies if the provided date is in a correct format and falls within the valid range of dates.
Problem statement
You are required to write a Python program that validates a given date and determines if it is a valid date or not.
Python Program to Validate a Date
def is_valid_date(date_string): try: # Split the date into day, month, and year day, month, year = map(int, date_string.split('/')) # Check if the year is within the valid range if year < 1 or year > 9999: return False # Check if the month is within the valid range if month < 1 or month > 12: return False # Check if the day is within the valid range for the given month and year if day < 1 or day > 31: return False # Check for specific month and day validity if month in [4, 6, 9, 11] and day > 30: return False if month == 2: if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0): if day > 29: return False elif day > 28: return False return True except ValueError: return False # Test the function date_input = input("Enter a date (in dd/mm/yyyy format): ") if is_valid_date(date_input): print("Valid date") else: print("Invalid date")
How it works
- The
is_valid_date()
function takes adate_string
as input, which represents the date in the format “dd/mm/yyyy”. - The function attempts to split the date string into day, month, and year by using the
split()
method and converting the resulting parts to integers. - It first checks if the year is within the valid range of 1 to 9999. If not, it returns
False
. - It then checks if the month is within the valid range of 1 to 12. If not, it returns
False
. - Next, it checks if the day is within the valid range of 1 to 31. If not, it returns
False
. - For months with only 30 days (April, June, September, November), it checks if the day is greater than 30 and returns
False
if it is. - For February, it checks if the year is a leap year (divisible by 4 and not divisible by 100, except if divisible by 400). If it is a leap year, it checks if the day is greater than 29 and returns
False
if it is. If it is not a leap year, it checks if the day is greater than 28 and returnsFalse
if it is. - If none of the above conditions are met, it returns
True
, indicating that the date is valid. - Finally, the program prompts the user to enter a date in the “dd/mm/yyyy” format and calls the
is_valid_date()
function to check if it is a valid date. It prints the corresponding message based on the validation result.
Input / Output
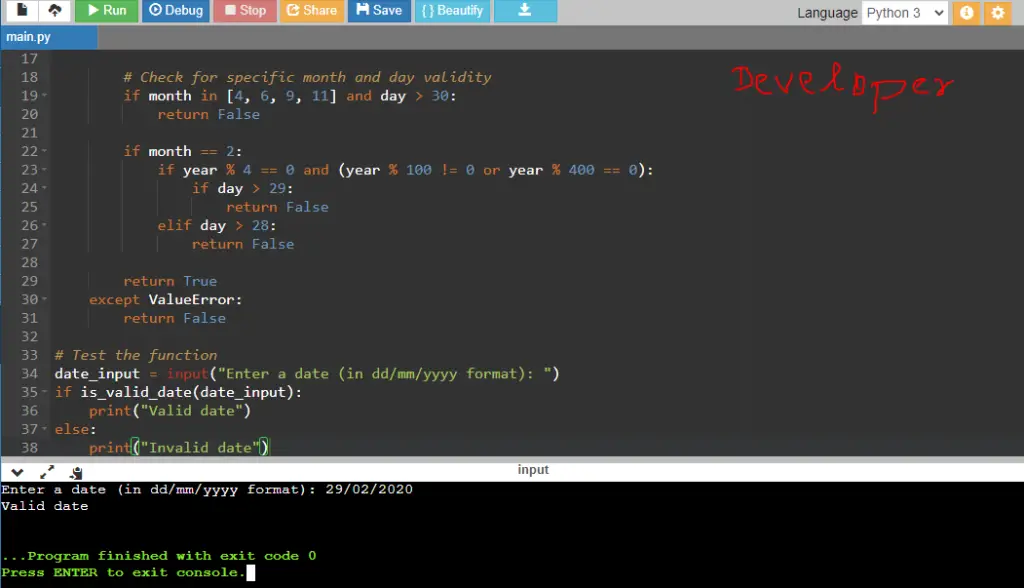