This Python program sorts a list of elements based on their lengths in ascending order. It demonstrates how to use the sorted()
function with a custom sorting key to achieve this.
Problem Statement
Given a list of strings, you need to sort the list in ascending order based on the length of the strings.
Python Program to Sort a List According to the Length of the Elements
def sort_by_length(input_list): sorted_list = sorted(input_list, key=len) return sorted_list # Input input_list = ["apple", "banana", "kiwi", "grapes", "orange", "strawberry"] # Sorting by length sorted_result = sort_by_length(input_list) # Output print("Original List:", input_list) print("Sorted List by Length:", sorted_result)
How It Works
- Define a function
sort_by_length(input_list)
that takes a list of strings as input. - Inside the function, use the
sorted()
function to sort the input list based on thelen
function as the sorting key. This means that the elements will be sorted based on their lengths. - Return the sorted list.
- Outside the function, create an example input list of strings.
- Call the
sort_by_length()
function with the input list and store the sorted result. - Print both the original input list and the sorted list based on length.
Input/Output
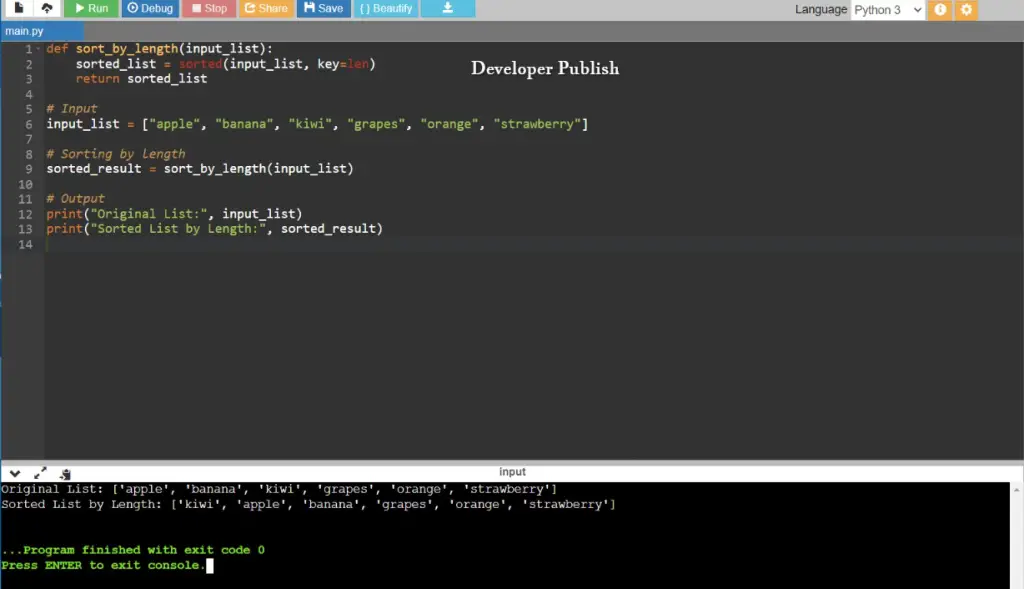