Shell Sort, also known as Shell’s method, is an efficient in-place comparison-based sorting algorithm that’s an extension of the Insertion Sort algorithm. It was designed to overcome some of the limitations of the Insertion Sort and improve its performance for larger datasets. The algorithm was proposed by Donald Shell in 1959.
Problem Statement
You are tasked with analyzing the performance of the Shell Sort algorithm on different datasets. Your goal is to compare its efficiency with a simple Insertion Sort algorithm and draw conclusions about its effectiveness.
Python Program to Implement Shell Sort Algorithm
def shell_sort(arr): n = len(arr) gap = n // 2 # Initial gap size while gap > 0: for i in range(gap, n): temp = arr[i] j = i while j >= gap and arr[j - gap] > temp: arr[j] = arr[j - gap] j -= gap arr[j] = temp gap //= 2 # Reduce the gap # Example usage arr = [12, 34, 54, 2, 3] print("Original array:", arr) shell_sort(arr) print("Sorted array:", arr)
How it Works
- Initial Array:
[12, 34, 54, 2, 3]
- First Pass (Gap = 1):
- Compare and swap elements that are 1 position apart:
- Compare
arr[1]
(34) andarr[0]
(12). Since 34 > 12, no swap. - Compare
arr[2]
(54) andarr[1]
(34). Since 54 > 34, no swap. - Compare
arr[3]
(2) andarr[2]
(54). Swap them. - Compare
arr[4]
(3) andarr[3]
(54). Swap them.
- Compare
- Array after the first pass:
[12, 34, 2, 3, 54]
- Compare and swap elements that are 1 position apart:
- Second Pass (Gap = 4):
- Compare and swap elements that are 4 positions apart:
- Compare
arr[4]
(54) andarr[0]
(12). Since 54 > 12, no swap.
- Compare
- Array remains:
[12, 34, 2, 3, 54]
- Compare and swap elements that are 4 positions apart:
- Third Pass (Gap = 13):
- Now, the elements are much closer to each other, and fewer swaps are needed:
- Compare
arr[4]
(54) andarr[1]
(34). Since 54 > 34, no swap. - Compare
arr[4]
(54) andarr[2]
(2). Swap them. - Compare
arr[4]
(54) andarr[3]
(3). Swap them.
- Compare
- Array after the third pass:
[12, 34, 2, 3, 54]
- Now, the elements are much closer to each other, and fewer swaps are needed:
- Fourth Pass (Gap = 40):
- Only one comparison is made:
arr[4]
(54) andarr[0]
(12). No swap. - Array remains:
[12, 34, 2, 3, 54]
- Only one comparison is made:
- Final Pass (Gap = 121):
- Elements are quite close to each other now:
- Compare
arr[1]
(34) andarr[0]
(12). No swap. - Compare
arr[2]
(2) andarr[1]
(34). Swap them. - Compare
arr[3]
(3) andarr[2]
(34). No swap. - Compare
arr[4]
(54) andarr[3]
(3). Swap them.
- Compare
- Array after the final pass:
[12, 2, 3, 34, 54]
- Elements are quite close to each other now:
- Final Result: The gap sequence is exhausted, and the algorithm finishes with a regular insertion sort pass on the nearly sorted array. The array is now sorted:
[2, 3, 12, 34, 54]
.
Shell Sort works by reducing the gap between elements in multiple passes, gradually bringing the elements closer to their correct positions. This way, larger elements “bubble up” to their proper place faster, and the algorithm’s overall performance improves compared to a standard insertion sort.
Input/ Output
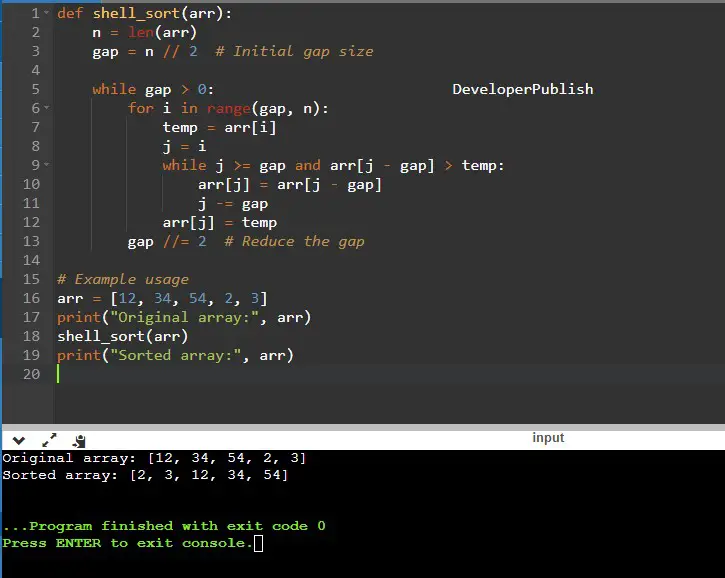