In this Python program, we will implement a recursive function to reverse a string. Recursion is a technique where a function calls itself to solve a smaller version of the problem. We will use this approach to reverse a given string.
Problem Statement
Given a string, we need to write a recursive function to reverse the string.
Python Program to Reverse a String using Recursion
def reverse_string(string): if len(string) == 0: return string else: return reverse_string(string[1:]) + string[0] # Test the function input_string = input("Enter a string: ") reversed_string = reverse_string(input_string) print("Reversed string:", reversed_string)
How its work
- The
reverse_string
function takes a string as input. - In the base case, if the length of the string is 0, we return the string as it is (empty string).
- In the recursive case, we recursively call the
reverse_string
function on a smaller version of the string, obtained by excluding the first character, and then append the first character at the end. - The function keeps calling itself until the length of the string becomes 0.
- Finally, the reversed string is returned.
Output
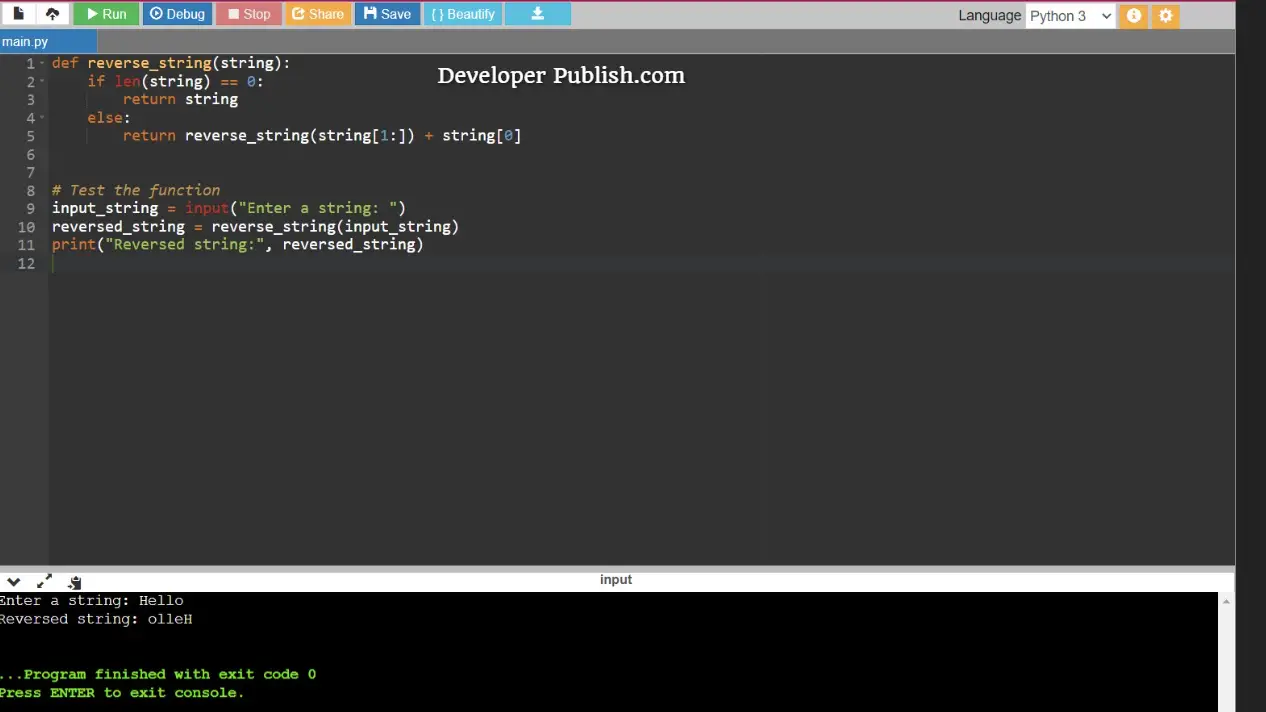