In this program, we will write a Python program to remove the odd-indexed characters from a given string.
Problem statement
Given a string, we need to remove all the characters at odd indices and return the resulting string.
Python Program to Remove Odd Indexed Characters in a string
def remove_odd_indexed_chars(string): result = "" for index in range(len(string)): if index % 2 == 0: result += string[index] return result # Test the program input_string = input("Enter a string: ") result_string = remove_odd_indexed_chars(input_string) print("Result:", result_string)
How it works
- We define a function
remove_odd_indexed_chars
that takes a string as an input. - We initialize an empty string
result
to store the characters at even indices. - We iterate over the range of indices of the string using a for loop and the
range
function. - Inside the loop, we check if the current index is even by using the modulo operator
%
. If the index is even (i.e.,index % 2 == 0
), we add the character at that index to theresult
string using string concatenation. - Finally, we return the
result
string, which contains only the characters at even indices.
Input / Output
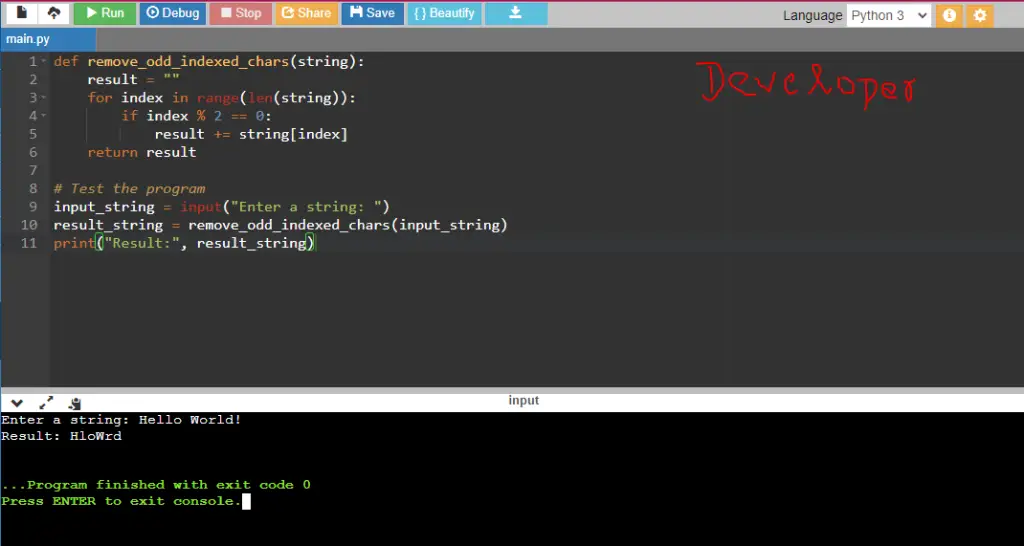