Python provides various methods for file operations, including reading and writing. This program demonstrates how to create a Python program that reads and displays the contents of a file.
Problem Statement
Write a Python program that takes the name of a file as input and reads and displays its contents.
Python Program to Read the Contents of the File
def read_file_contents(filename): try: with open(filename, 'r') as file: contents = file.read() return contents except FileNotFoundError: return "File not found." # Input file_name = input("Enter the name of the file: ") # Reading file contents file_contents = read_file_contents(file_name) # Output print("Contents of the file:") print(file_contents)
Input / Output
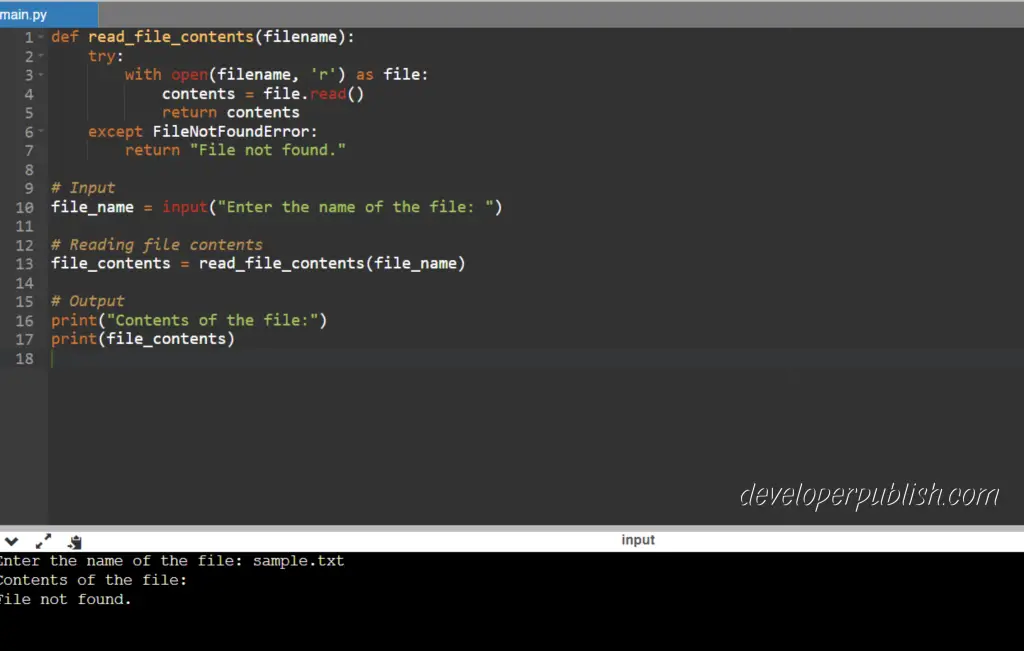