Appending data to a file is a common operation in programming, especially when you want to store data for later use or maintain a record of inputs. In this context, let’s break down the process of reading a string from the user and appending it to a file using Python.
Problem Statement
You are required to write a Python program that reads a string input from the user and appends it to a file named “output.txt”. Each string entered by the user should be written on a new line in the file. The program should ensure that the file is properly managed, opened in append mode, and closed after writing the input string.
Python Program to Read a String from the User and Append it into a File
def main(): # Get the input string from the user input_string = input("Enter a string: ") # Open the file in append mode and write the input string with open("output.txt", "a") as file: file.write(input_string + "\n") print("String appended to the file.") if __name__ == "__main__": main()
How it Works
- Function Definition: The program starts by defining a function named
main()
. This is a common practice in Python to encapsulate the main logic of the program within a function. - User Input: The
input()
function is used to prompt the user for input. The text “Enter a string: ” is displayed on the screen, and the user can type in their desired string. The input string is then stored in theinput_string
variable. - File Handling: The
with
statement is used to open the file named “output.txt” in append mode ("a"
). This means that if the file exists, the program will add new content to the end of the file. If the file doesn’t exist, it will be created. The file is opened and managed within the context of thewith
block.Inside the block, thefile.write()
method is used to write theinput_string
to the file. The+ "\n"
adds a newline character at the end of the string to ensure that each input string is written on a new line. - File Closure: The
with
statement automatically takes care of closing the file once the block is exited. This is important to ensure that the file resources are properly managed and released. - Final Output: After writing the string to the file, a confirmation message is printed to the screen.
- Main Execution: This block of code checks whether the script is being run as the main program (not imported as a module). If it is the main program, the
main()
function is called, and the program’s logic is executed.
Input/ Output
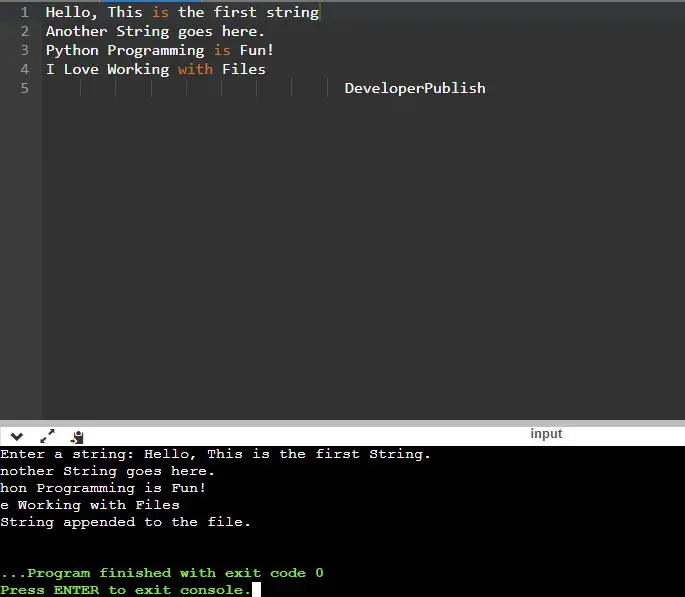