In this python program, we will write a recursive Python function to print the binary equivalent of an integer. Recursion is a technique where a function calls itself repeatedly until a specific condition is met.
Problem statement
Write a Python program to print the binary equivalent of a given integer using recursion.
Python Program to Print Binary Equivalent of an Integer using Recursion
def binary_equivalent(num): if num == 0: return '' else: return binary_equivalent(num // 2) + str(num % 2) # Get input from the user num = int(input("Enter an integer: ")) # Call the function and print the result print("Binary equivalent:", binary_equivalent(num))
How it works
- Define a recursive function, let’s call it
binary_equivalent
, which takes an integernum
as input. - Inside the function, check if
num
is equal to 0. If it is, return an empty string as the base case. - Otherwise, recursively call the
binary_equivalent
function withnum
divided by 2 and concatenate the remainder ofnum
divided by 2 with the recursive call. - Finally, return the binary representation obtained from the recursive call.
Input / output
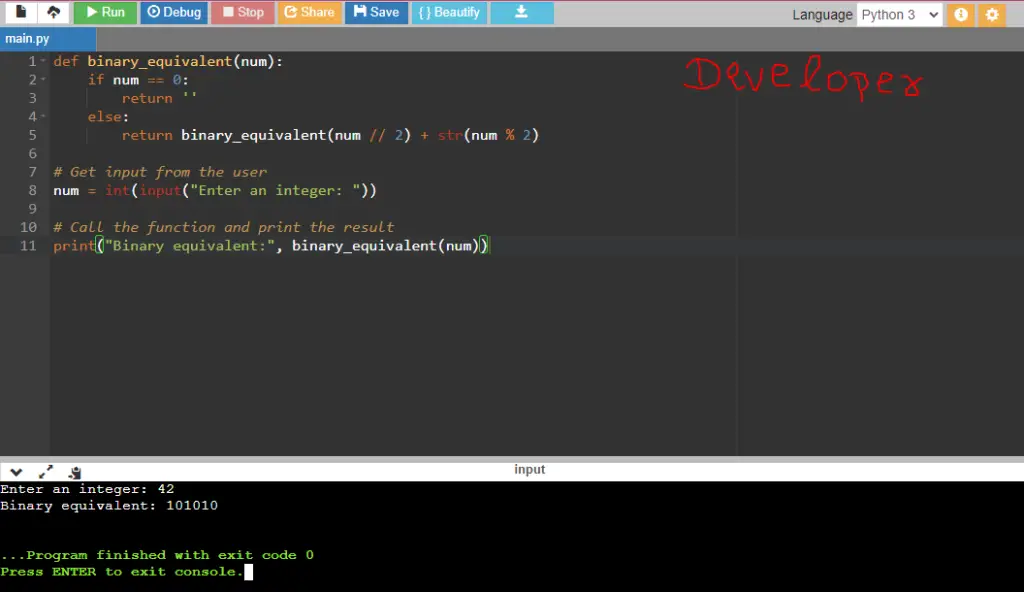