In this Python program, we will demonstrate how to combine two lists into one and then sort the resulting merged list in ascending order. This can be particularly useful when you have two separate datasets or lists of elements that you want to combine and arrange in a specific order.
Problem statement
Write a Python program that takes two lists as input, merges them into a single list, and then sorts the merged list in ascending order. Your program should display the sorted merged list as the final output.
Python Program to Merge Two Lists and Sort it
# Function to merge and sort two lists def merge_and_sort_lists(list1, list2): # Merge the two lists merged_list = list1 + list2 # Sort the merged list sorted_merged_list = sorted(merged_list) return sorted_merged_list # Example lists list1 = [5, 2, 9, 1] list2 = [7, 3, 8, 4] # Merge and sort the lists result = merge_and_sort_lists(list1, list2) # Print the sorted merged list print("Merged and sorted list:", result)
How it works
Here’s a step-by-step explanation of how the Python program to merge two lists and sort them works:
- Initialization:
- Two lists,
list1
andlist2
, are defined. These lists contain integer elements.
- Two lists,
list1 = [5, 2, 9, 1]
list2 = [7, 3, 8, 4]
2. Merging the Lists:
- The
+
operator is used to mergelist1
andlist2
into a single list calledmerged_list
.
Code:
merged_list = list1 + list2
- After this step,
merged_list
will contain all the elements fromlist1
followed by all the elements fromlist2
. In this case,merged_list
will be[5, 2, 9, 1, 7, 3, 8, 4]
.
3. Sorting the Merged List:
- The
sorted()
function is applied tomerged_list
. This function sorts the elements ofmerged_list
in ascending order.
Code:
sorted_merged_list = sorted(merged_list)
After this step, sorted_merged_list
will contain the elements of merged_list
sorted in ascending order. In this case, sorted_merged_list
will be [1, 2, 3, 4, 5, 7, 8, 9]
.
4. Printing the Result:
- The program prints the sorted merged list as the final output.
code:
print(“Merged and sorted list:”, sorted_merged_list)
The output will be:
Merged and sorted list: [1, 2, 3, 4, 5, 7, 8, 9]
So, in summary, this program takes two lists, merges them into one, and then sorts the merged list in ascending order. The result is printed as the sorted merged list. You can replace list1
and list2
with different lists to merge and sort as needed.
Input/Output
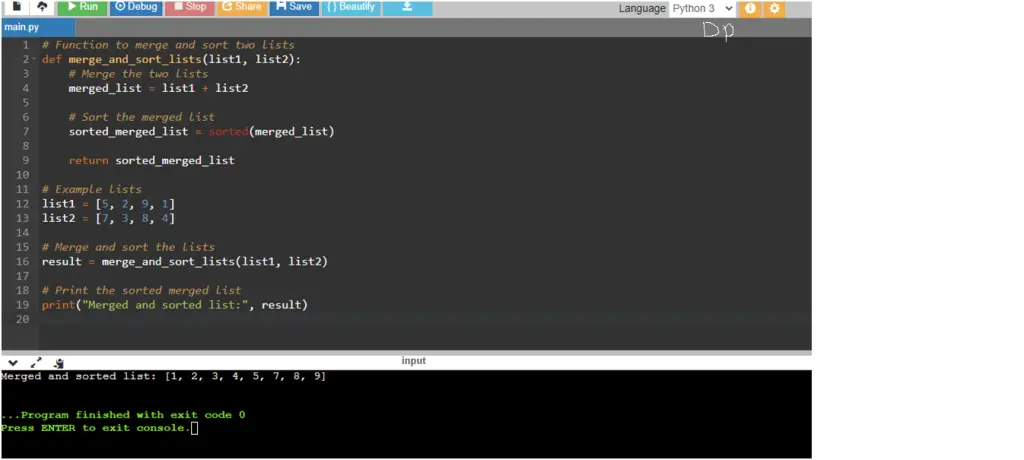