In this Python program, we’ll delve into the implementation of a stack data structure using a linked list. Stacks are essential in various computing applications, and understanding this implementation will provide insights into combining linked lists to create a functional stack.
Problem statement
The task is to create a Python program that demonstrates the implementation of a stack using a linked list. This program will help you comprehend the basic principles of stacks and linked lists.
Python Program to Implement Stack using Linked List
class Node: def __init__(self, data): self.data = data self.next = None class Stack: def __init__(self): self.top = None def push(self, data): new_node = Node(data) if not self.top: self.top = new_node else: new_node.next = self.top self.top = new_node def pop(self): if not self.top: return None popped = self.top.data self.top = self.top.next return popped def peek(self): return self.top.data if self.top else None def is_empty(self): return self.top is None # Example usage if __name__ == "__main__": stack = Stack() stack.push(10) stack.push(20) stack.push(30) print("Top element:", stack.peek()) print("Popped element:", stack.pop()) print("Is the stack empty?", stack.is_empty())
Input / Output
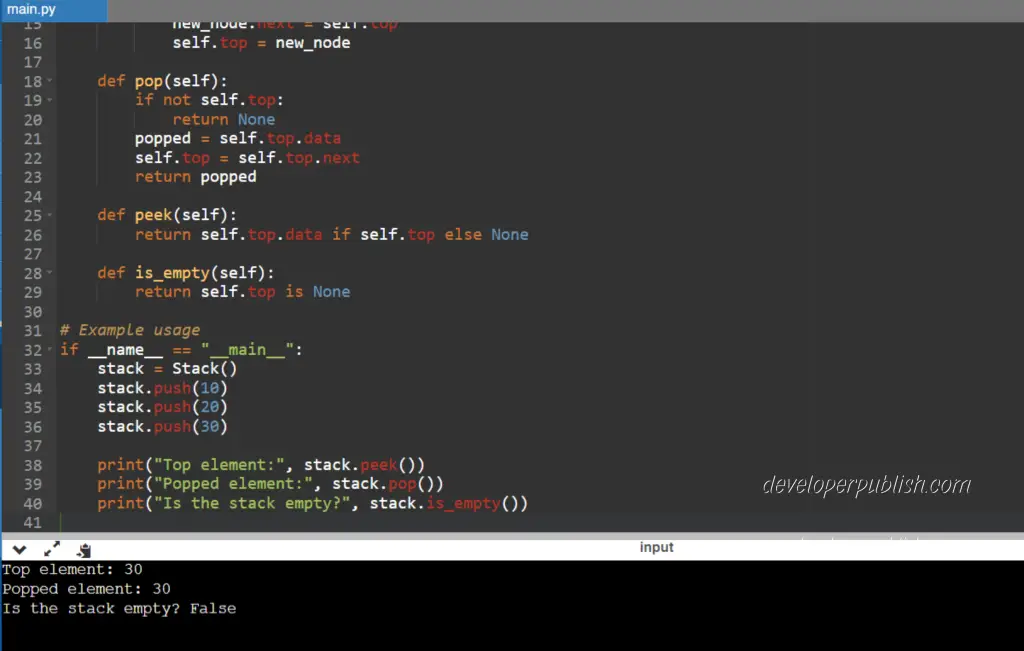