Finding the union of two lists is a common operation in programming, particularly when dealing with data manipulation and set operations. The union of two lists refers to the operation of combining both lists to create a new list that contains all unique elements from both lists, without any duplicates. In other words, the union of two lists contains all distinct elements present in either or both of the original lists.
Problem Statement
You are given two lists, List A and List B, containing integer elements. Your task is to write a python program that finds the union of these two lists. The union of two lists is defined as a new list that contains all unique elements from both List A and List B, without any duplicates.
Python Program to Find the Union of Two Lists
def find_union(list1, list2): union = list(set(list1) | set(list2)) return union # Example lists list1 = [1, 2, 3, 4, 5] list2 = [4, 5, 6, 7, 8] union_result = find_union(list1, list2) print("Union of the two lists:", union_result)
How it Works
- Function Definition (
find_union
):- The function
find_union
is defined to take two lists as parameters:list1
andlist2
.
- The function
- Conversion to Sets:
- Inside the function, both
list1
andlist2
are converted into sets using theset()
function. This step removes any duplicate elements from each list, as sets do not allow duplicates.
- Inside the function, both
- Union Operation:
- The
|
(pipe) operator is used to perform a union operation between the two sets obtained fromlist1
andlist2
. This operation combines the elements of both sets while discarding duplicate values.
- The
- Conversion Back to List:
- The resulting set from the union operation is then converted back into a list using the
list()
constructor. This step is necessary to present the final result as a list.
- The resulting set from the union operation is then converted back into a list using the
- Output:
- The
union
list, which now contains all the unique elements from bothlist1
andlist2
, is returned from the function. - The program then assigns the returned list to the variable
union_result
.
- The
- Print Result:
- Finally, the program prints the contents of the
union_result
list, which is the union of the two input lists, in the following format: “Union of the two lists: [1, 2, 3, 4, 5, 6, 7, 8]”.
- Finally, the program prints the contents of the
Input/ Output
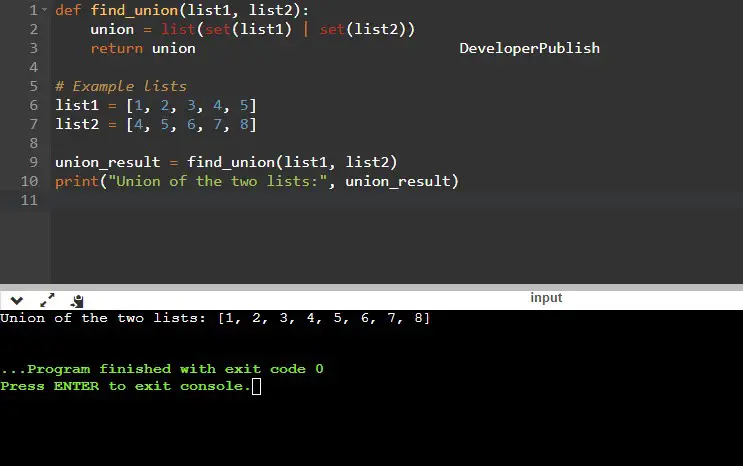