In this Python program, we’ll create a program to find the sum of all the values in a dictionary. Given a dictionary with numeric values, the program will calculate and display the sum of those values.
Problem Statement:
Given a dictionary containing numeric values, we want to calculate the sum of all the values in the dictionary.
Python Program to Find the Sum of All the Items in a Dictionary
def calculate_sum(dictionary): total_sum = sum(dictionary.values()) return total_sum def main(): data = { 'item1': 10, 'item2': 20, 'item3': 30, 'item4': 40, 'item5': 50 } result = calculate_sum(data) print("Sum of all items:", result) if __name__ == "__main__": main()
How it Works:
- We define a function
calculate_sum
that takes a dictionary as its parameter. - Inside the
calculate_sum
function, we use thesum()
function along with thevalues()
method of the dictionary to calculate the sum of all the values in the dictionary. - The
main()
function contains a sample dictionary nameddata
with numeric values. - We call the
calculate_sum()
function with thedata
dictionary as an argument and store the result in theresult
variable. - Finally, we print out the sum of all the items in the dictionary.
Input/Output:
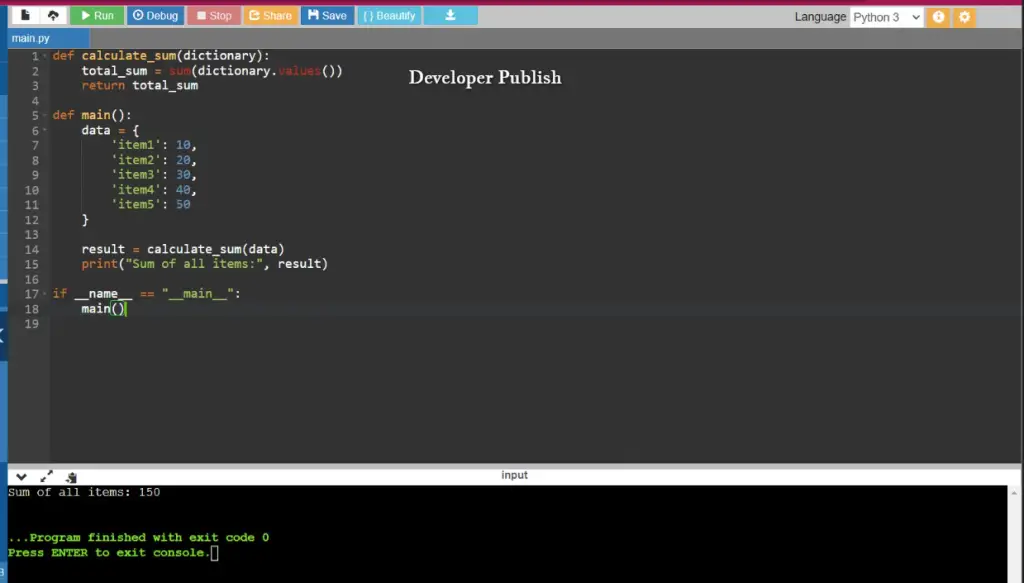