This Python program aims to find the smallest divisor of a given integer. It prompts the user to enter an integer, calculates the smallest divisor using a function, and displays the result on the console.
Problem Statement
Write a Python program to find the smallest divisor of a given integer.
Python Program to Find the Smallest Divisor of an Integer
def smallest_divisor(num): """ Finds the smallest divisor of a given integer. Args: num (int): The integer for which the smallest divisor needs to be found. Returns: int: The smallest divisor of the given integer. """ # Checking if 1 is a divisor if num > 1: return 1 # Finding the smallest divisor for i in range(2, int(num ** 0.5) + 1): if num % i == 0: return i # If no divisor is found, the number is prime return num # Prompting the user to enter an integer num = int(input("Enter an integer: ")) # Finding the smallest divisor result = smallest_divisor(num) # Displaying the result print("The smallest divisor of {} is: {}".format(num, result))
How it works
- The function
smallest_divisor
takes an integernum
as input and returns the smallest divisor ofnum
. - The function checks if the input
num
is greater than 1. If it is, then 1 is returned since it is the smallest divisor of any number. - Next, a loop is executed from 2 to the square root of
num
(inclusive). This loop checks ifnum
is divisible by any number in that range. - If a divisor is found, that divisor is returned as the smallest divisor.
- If no divisor is found in the loop, it means the number is prime, and
num
itself is returned as the smallest divisor. - The program prompts the user to enter an integer and stores it in the variable
num
. - It then calls the
smallest_divisor
function withnum
as the argument, and the returned value is stored in the variableresult
. - Finally, the program displays the result by printing the smallest divisor using the
print
statement.
Input / Output
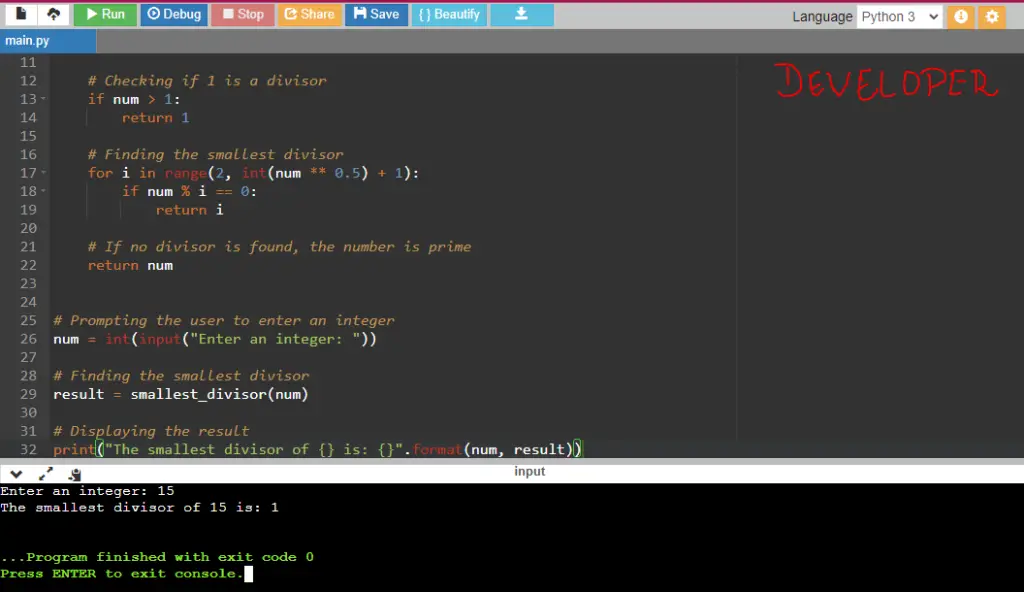