In this Python program, we will create a class to calculate the area of a rectangle. We’ll define a class called Rectangle
with methods to set the dimensions of the rectangle and calculate its area.
Problem Statement:
Write a Python program to find the area of a rectangle using classes. The program should take the length and width of the rectangle as input and output the calculated area.
Python Program to Find the Area of a Rectangle using Classes
class Rectangle: def __init__(self): self.length = 0 self.width = 0 def set_dimensions(self, length, width): self.length = length self.width = width def calculate_area(self): return self.length * self.width def main(): rectangle = Rectangle() length = float(input("Enter the length of the rectangle: ")) width = float(input("Enter the width of the rectangle: ")) rectangle.set_dimensions(length, width) area = rectangle.calculate_area() print(f"The area of the rectangle is: {area}") if __name__ == "__main__": main()
How it Works:
- We start by defining a class called
Rectangle
with an__init__
method to initialize the length and width to zero. - The class has two methods,
set_dimensions
andcalculate_area
.set_dimensions
takes the length and width as arguments and assigns them to the respective attributes of the class.calculate_area
calculates the area of the rectangle using the formulalength * width
and returns the result.
- In the
main
function, we create an instance of theRectangle
class. - We take user input for the length and width of the rectangle.
- We call the
set_dimensions
method to set the dimensions of the rectangle. - The
calculate_area
method is then called to calculate the area of the rectangle. - Finally, the calculated area is printed.
Input/Output:
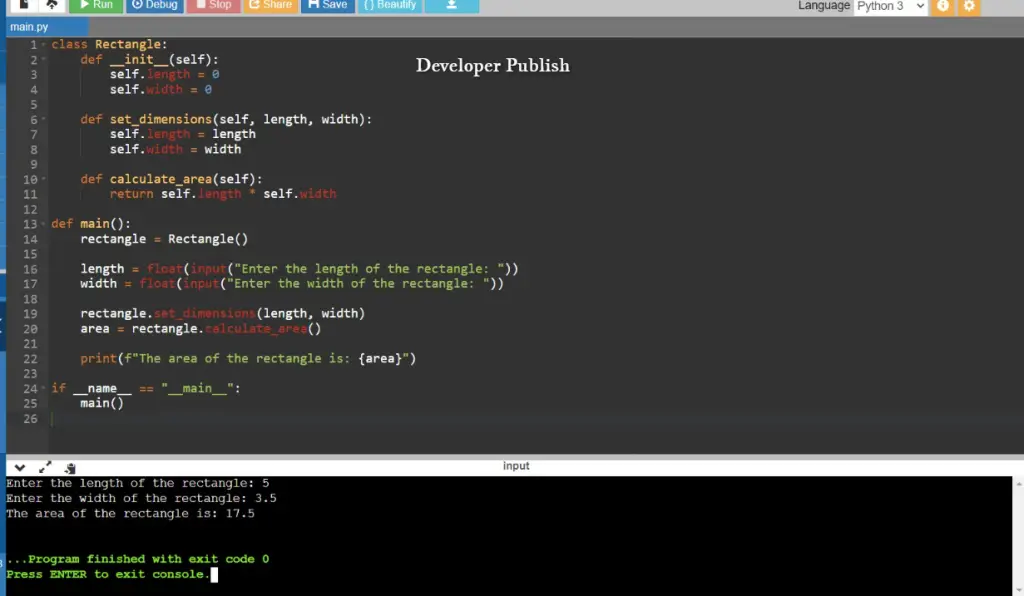