This Python program finds and displays the common characters present in two input strings.
Problem Statement:
Given two strings, we need to write a program that identifies and outputs the characters that are common in both strings.
Python Program to Find Common Characters in Two Strings
def find_common_characters(str1, str2): common_chars = set() for char in str1: if char in str2: common_chars.add(char) return common_chars # Input strings input_str1 = input("Enter the first string: ") input_str2 = input("Enter the second string: ") common_characters = find_common_characters(input_str1, input_str2) if common_characters: print("Common characters:", ', '.join(common_characters)) else: print("No common characters found.")
How It Works:
- The program defines a function
find_common_characters
that takes two input stringsstr1
andstr2
as arguments. - It initializes an empty set called
common_chars
to store the common characters found. - The program iterates through each character in
str1
. If the character is also present instr2
, it adds the character to thecommon_chars
set. - After iterating through all characters in
str1
, the program returns the set of common characters. - The program then takes user input for two strings,
input_str1
andinput_str2
. - It calls the
find_common_characters
function with the input strings and stores the result in thecommon_characters
variable. - If there are common characters, the program prints them out, separated by commas. If no common characters are found, it prints a message indicating that.
Input/Output:
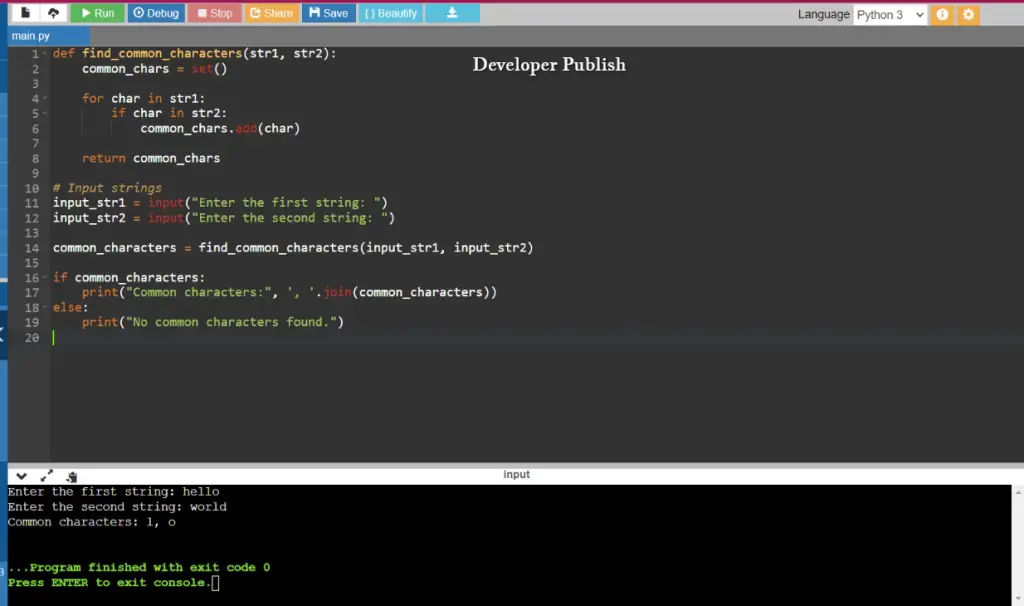