A circular singly linked list is a variant of the linked list data structure in which the last node points back to the first node, forming a loop. This Python program demonstrates the implementation and various operations on a circular singly linked list using Python.
Problem statement
You are required to implement a circular singly linked list that supports two primary operations: appending elements to the list and displaying the elements in order. The circular aspect means that the last node’s ‘next’ pointer points back to the first node, forming a continuous loop.
Python Program to Demonstrate Circular Single Linked List
class Node: def __init__(self, data): self.data = data self.next = None class CircularLinkedList: def __init__(self): self.head = None def append(self, data): new_node = Node(data) if not self.head: self.head = new_node new_node.next = self.head else: current = self.head while current.next != self.head: current = current.next current.next = new_node new_node.next = self.head def display(self): if not self.head: print("List is empty") return current = self.head while True: print(current.data, end=" -> ") current = current.next if current == self.head: break print("...") # Input: Creating a circular singly linked list circular_list = CircularLinkedList() elements = [1, 2, 3, 4, 5] for element in elements: circular_list.append(element) print("Circular Linked List:") circular_list.display()
Input / Output
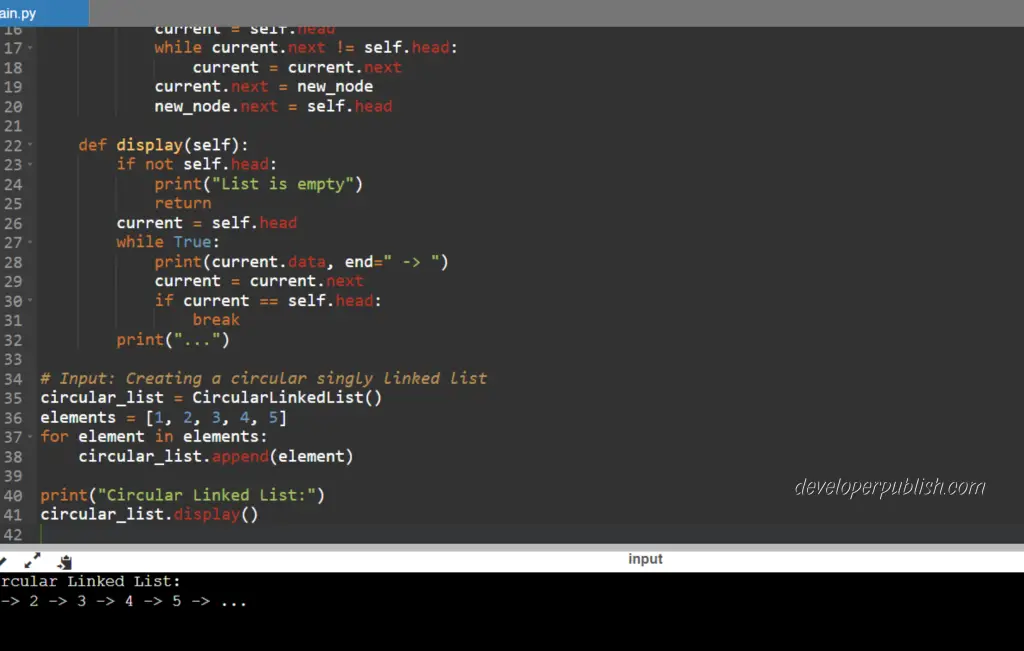