In Python, objects can have attributes that store various types of data. This program illustrates how to create a Python program that converts an object’s attributes into a dictionary.
Problem Statement
Write a Python program that takes an object as input and creates a dictionary containing its attributes as key-value pairs.
Python Program to Create Dictionary from an Object
class Person: def __init__(self, name, age, city): self.name = name self.age = age self.city = city def object_to_dictionary(obj): obj_dict = {} for attribute in dir(obj): if not attribute.startswith("__") and not callable(getattr(obj, attribute)): obj_dict[attribute] = getattr(obj, attribute) return obj_dict # Input (Creating an object) name = input("Enter the person's name: ") age = int(input("Enter the person's age: ")) city = input("Enter the person's city: ") person_object = Person(name, age, city) # Converting object to dictionary person_dict = object_to_dictionary(person_object) # Output print("Dictionary representation of the object:", person_dict)
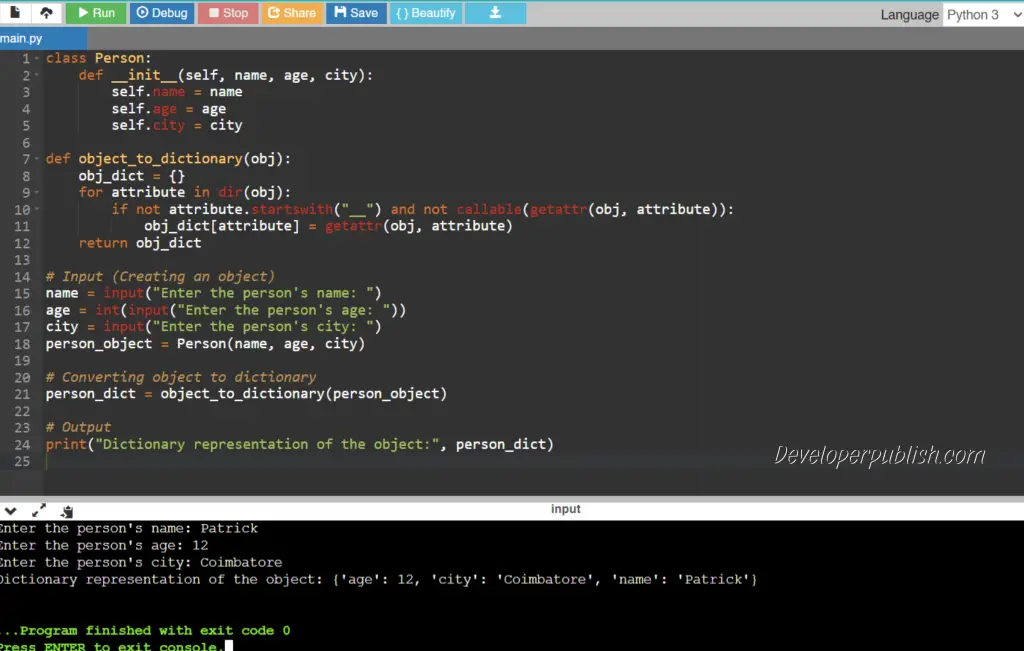