In this Python program, we will convert Gray codes to binary codes. Gray code is a binary numeral system where two successive values differ by only one bit. Converting Gray codes to binary codes allows us to obtain the corresponding binary representation.
Problem Statement
Given a Gray code as input, we need to convert it to its equivalent binary code.
Python Program to Convert Gray to Binary Code
def gray_to_binary(gray_code): binary_code = gray_code[0] for i in range(1, len(gray_code)): if gray_code[i] == '0': binary_code += binary_code[i-1] else: binary_code += str(1 - int(binary_code[i-1])) return binary_code # Test the program gray_code = input("Enter the Gray code: ") binary_code = gray_to_binary(gray_code) print(f"Binary code: {binary_code}")
How It Works
- The function
gray_to_binary(gray_code)
takes a Gray code as input and returns its equivalent binary code. - We initialize
binary_code
as the first character of the Gray code, as it remains unchanged during conversion. - Starting from the second character of the Gray code, we iterate through each bit.
- If the current bit is ‘0’, we append the same value as the previous bit of
binary_code
tobinary_code
. - If the current bit is ‘1’, we append the complement of the previous bit of
binary_code
tobinary_code
(1 minus the previous bit value). - After iterating through all the bits of the Gray code, we have the complete binary code.
- Finally, we return
binary_code
, which will be the equivalent binary code of the input Gray code.
Input/Output
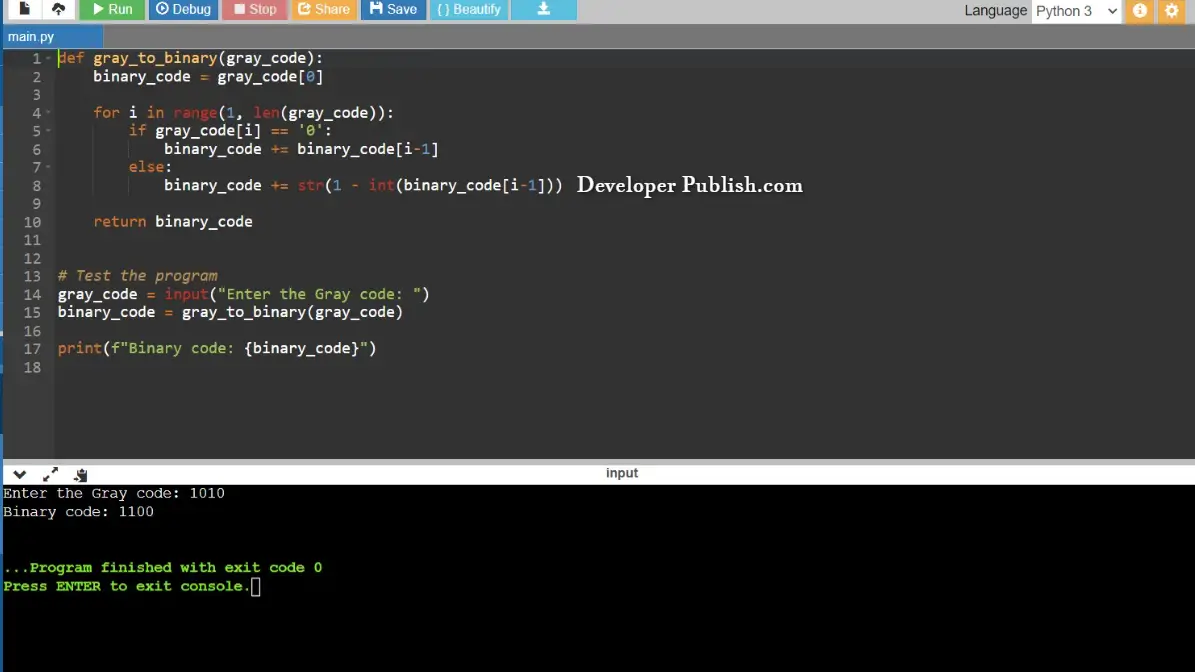