This Python program checks if two numbers are amicable numbers or not. Amicable numbers are pairs of numbers where the sum of the proper divisors of each number equals the other number.
Amicable numbers are a pair of numbers where the sum of the proper divisors of one number is equal to the other number, and vice versa. In other words, the aliquot sum (the sum of proper divisors excluding the number itself) of one number equals the other number.
Problem Statement
Write a Python program to determine if two numbers are amicable numbers or not. An amicable number is a pair of numbers (num1, num2) where the sum of the proper divisors of num1 equals num2, and the sum of the proper divisors of num2 equals num1.
Python Program to Check If Two Numbers are Amicable Numbers or Not
def get_proper_divisors(n): divisors = [] for i in range(1, n): if n % i == 0: divisors.append(i) return divisors def are_amicable_numbers(num1, num2): divisors_num1 = get_proper_divisors(num1) sum_num1 = sum(divisors_num1) divisors_num2 = get_proper_divisors(num2) sum_num2 = sum(divisors_num2) if sum_num1 == num2 and sum_num2 == num1: return True else: return False # Example usage num1 = int(input("Enter the first number: ")) num2 = int(input("Enter the second number: ")) if are_amicable_numbers(num1, num2): print(f"{num1} and {num2} are amicable numbers.") else: print(f"{num1} and {num2} are not amicable numbers.")
How it Works
- The program defines a function
get_proper_divisors(n)
that takes a numbern
as input and returns a list of its proper divisors. It iterates from 1 ton-1
and checks ifn
is divisible by the current number. If it is, the current number is added to the list of divisors. - The program defines another function
are_amicable_numbers(num1, num2)
that takes two numbersnum1
andnum2
as input. Inside this function:- It calls the
get_proper_divisors()
function fornum1
andnum2
to get their respective lists of proper divisors. - It calculates the sum of the proper divisors for
num1
and assigns it tosum_num1
, and calculates the sum of the proper divisors fornum2
and assigns it tosum_num2
. - It checks if
sum_num1
is equal tonum2
andsum_num2
is equal tonum1
. If both conditions are true, it returnsTrue
, indicating that the numbers are amicable. Otherwise, it returnsFalse
.
- It calls the
- The program prompts the user to enter two numbers,
num1
andnum2
, using theinput()
function. - It calls the
are_amicable_numbers()
function withnum1
andnum2
as arguments to check if they are amicable numbers. - If the returned value is
True
, the program displays a message stating that the numbers are amicable. Otherwise, it displays a message stating that the numbers are not amicable. - The program execution ends.
To check if two numbers are amicable, the program calculates the sum of the proper divisors for each number and compares it with the other number. If the sums match for both numbers, they are considered amicable. Otherwise, they are not amicable.
You can run the program and input different pairs of numbers to see if they are amicable or not.
Input/ Output
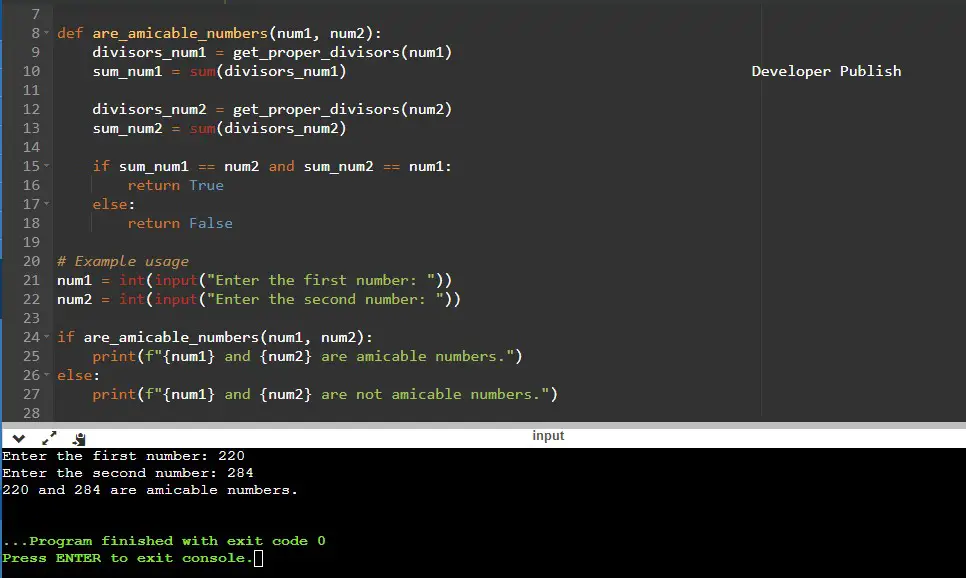
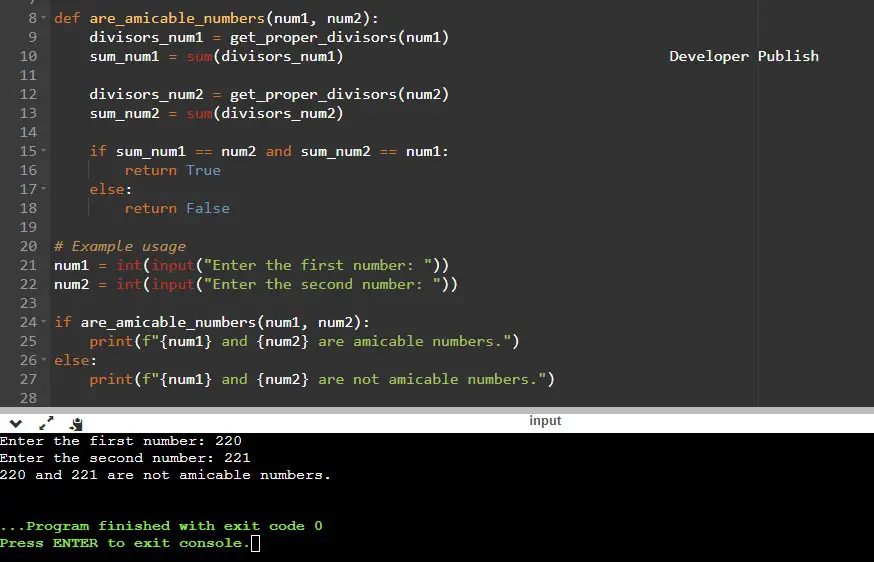