This python program demonstrates how to add a new key-value pair to an existing dictionary in Python.
Problem Statement:
You need to write a program that takes a key and a value as input and adds them as a new key-value pair to an existing dictionary.
Python Program to Add a Key-Value Pair to the Dictionary
def add_key_value(dictionary, key, value): dictionary[key] = value # Existing dictionary my_dictionary = {'a': 1, 'b': 2, 'c': 3} # Input new_key = input("Enter the new key: ") new_value = input("Enter the new value: ") # Adding the key-value pair add_key_value(my_dictionary, new_key, new_value) # Output print("Updated Dictionary:", my_dictionary)
Python
​x
16
1
def add_key_value(dictionary, key, value):
2
dictionary[key] = value
3
​
4
# Existing dictionary
5
my_dictionary = {'a': 1, 'b': 2, 'c': 3}
6
​
7
# Input
8
new_key = input("Enter the new key: ")
9
new_value = input("Enter the new value: ")
10
​
11
# Adding the key-value pair
12
add_key_value(my_dictionary, new_key, new_value)
13
​
14
# Output
15
print("Updated Dictionary:", my_dictionary)
16
​
How It Works:
- The
add_key_value
function takes three parameters:dictionary
,key
, andvalue
. - Inside the function, it adds the new key-value pair to the provided dictionary using the given key and value.
- The program starts with an existing dictionary named
my_dictionary
. - The user is prompted to input a new key and a new value.
- The
add_key_value
function is called to add the new key-value pair to themy_dictionary
. - Finally, the updated dictionary is printed.
Input/Output:
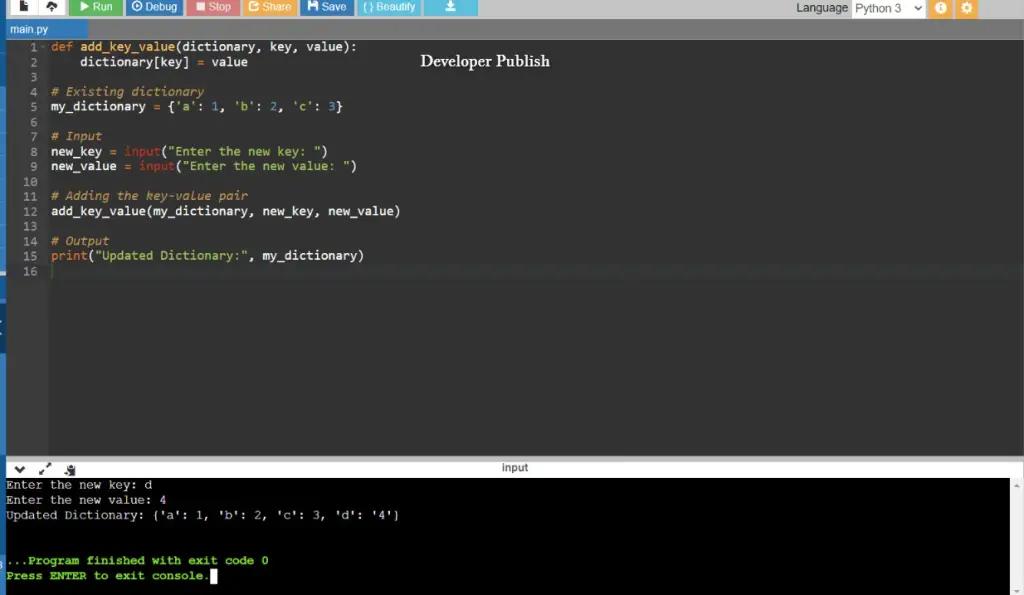