The Object Initializer and Collection Initializer in C# are similar to initialized Array and Object Constructor where we are able to set the properties of the newly created objects or multiple instances of the new object in essentially one line of code.
What is Object and Collection Initializer in C# ?
Object initializers are similar to the constructors that populates the properties of the newly created instances of the object.
Unlike the constructor,you can pick and choose which properties to populate in any combination .
class Address { public string street { get; set; } public string city { get; set; } } class Users { public int UserID { get; set; } public string UserName { get; set; } public string FirstName { get; set; } public Address ResidentialAddress { get; set; } public Users(int AUserID, string AUserName) { UserID = AUserID; UserName = AUserName; } }
When instantiating and using new classes,the standard techniques would be something like this.
Users user1 = new Users(); user1.FirstName = "senthil"; user1.UserID = 1; user1.UserName = "iamsenthil";
We can also use the technique of the overloaded constructor to initialize the new class object.
Users user1 = new Users(1,"senthil");
If you wanted to make this more cleaner with just one line code , this is the feature to look out for .
Users user1 = new Users { FirstName = "senthil", UserID = 1, UserName = "iamsenthil" };
Array Initialization
Say we want to create an array of integers
int[] numbers = { 42,10,20,45,60};
so we are able to declare the initializer with one line of code.
The object initializer borrows it syntax from the Array Initializer.
You will also notice that when i use the Object Initializer , i get the intellisense support that shows all the properties of the object.
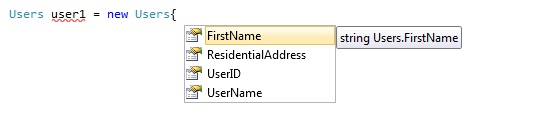
Users user1 = new Users { FirstName = "senthil", UserID = 1, UserName = "iamsenthil" };
This will implicitly call the default constructor ( with no arguments ) .
We can explicitly call the default constructor with this syntax.
Users user1 = new Users() { FirstName = "senthil", UserID = 1, UserName = "iamsenthil" };
The compiler will automatically generate the setter property code of the class.
We can also have the nested object initializer , one object initializer can contain creation of the new instances of the object that also is initialized in the same manner .
Eg :
Users user2 = new Users { ResidentialAddress = new Address { city = "Bangalore", street = "1234" } };
Note : We donot have to initialize all the properties , we can initialize some of them.
The same technique can be applied to the collection initializer too.Like the object initializer syntax that made the code look neater for objects, collection initializer syntax does the same for collections ..
They work with any type that implements the Generic ICollection of Type T.( ICollection interface)
List num = new List{12,56,78,23};
ArrayList objArray= new ArrayList{user1,user2,user3};