Anonymous Types in C# was a new feature that was introduced in C# 3.0 and this blog post explain what is it and how to use it in your C# application.
Anonymous types are on the fly classes or unnamed classes. For example , below is a sample code snippet showing how to define a class
class Employee { private int _EmpID; private string _EmpName; public int EmpID { get { return _EmpID; } set { _EmpID = value; } } public string EmpName { get { return _EmpName; } set { _EmpName = value; } } }
Here’s how to create an object
Employee objEmployee = new Employee { EmpID = 1, EmpName = "senthil" };
Note that the properties/ members are initialized here through object initializer. An object initializer can specify values for one or more properties of an object.
Class members such as methods or events are not allowed.
What are Anonymous Types in C# ?
We can also create an object via Implicit type reference .
var objEmployee = new Employee { EmpID = 1, EmpName = "senthil" };
Here’s how i use the Anonymous type
var objEmployee1 = new { EmpID = 1, EmpName = "senthil" };
The actual type of this would be anonymous . It has no name. This feature will be extremely be helpful when LINQ is used .
The Visual Studio will provide us the full intellisense support for the anonymous type along with the compile time checking.
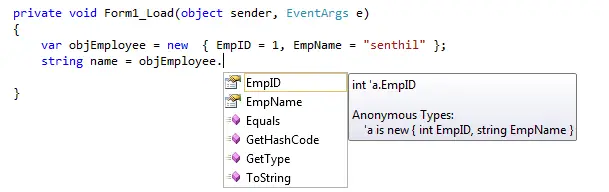
Little observations on the anonymous type .
1. I created another anonymous object with the same parameter and when I compare the type of objEmployee1 , objEmployee2 , they are same in the eyes of the compiler .
var objEmployee2 = new { EmpID = 6, EmpName = "trivium" };
2. I tried changing the order of the parameters of objuEmployee2.
var objEmployee2 = new { EmpName = "trivium",EmpID = 6 };
The type name here I got were different .
1 Comment