In this post, you’ll be learning about Conditional statements in Excel VBA and how you can use them in your Excel spreadsheets. Â
Conditional Statements in Excel VBA
Conditional Statements in VBA is used to make decisions between the given options or to make decision based on a set of conditions.
The following conditional statements are in VBA
If Statement
If statement is a conditional statement in VBA used when a particular condition needs to be satisfied.
Syntax
 IF condition Then true_code [Else false_code]
Sample program using IF statement in VBA
Input
Sub CheckScore() If Range("A1").Value >=35 Then MsgBox "Pass" End Sub
Output
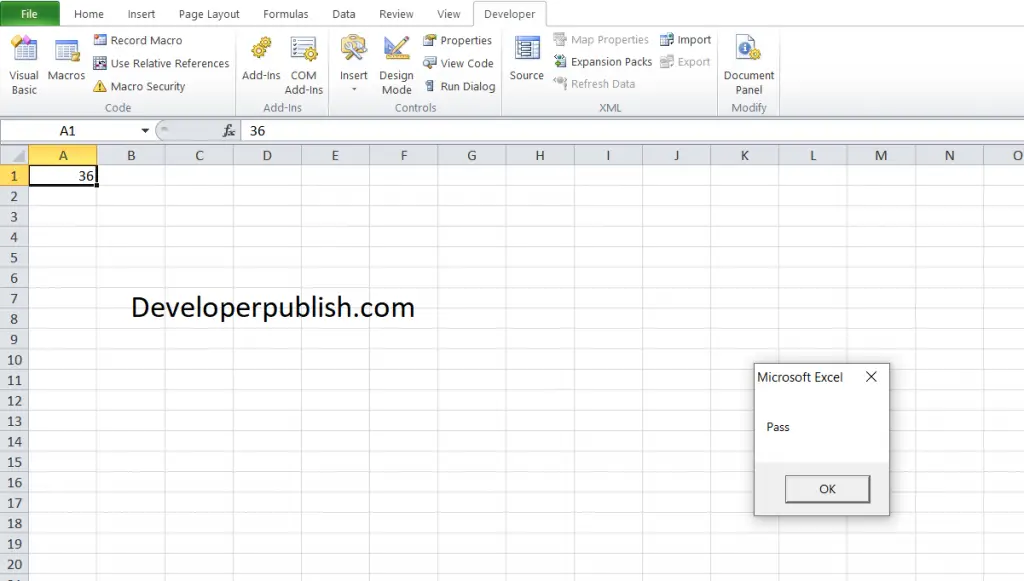
If Else
This VBA conditional statement is used when a decision is need to be made between two conditions
Syntax
IF condition Then true_code Else false_code End IF
Sample program using IF else statement in VBA
Input
Sub CheckScore() If Range("A1").Value >= 35 Then MsgBox "Pass" Else MsgBox "Fail" End If End Sub
Output
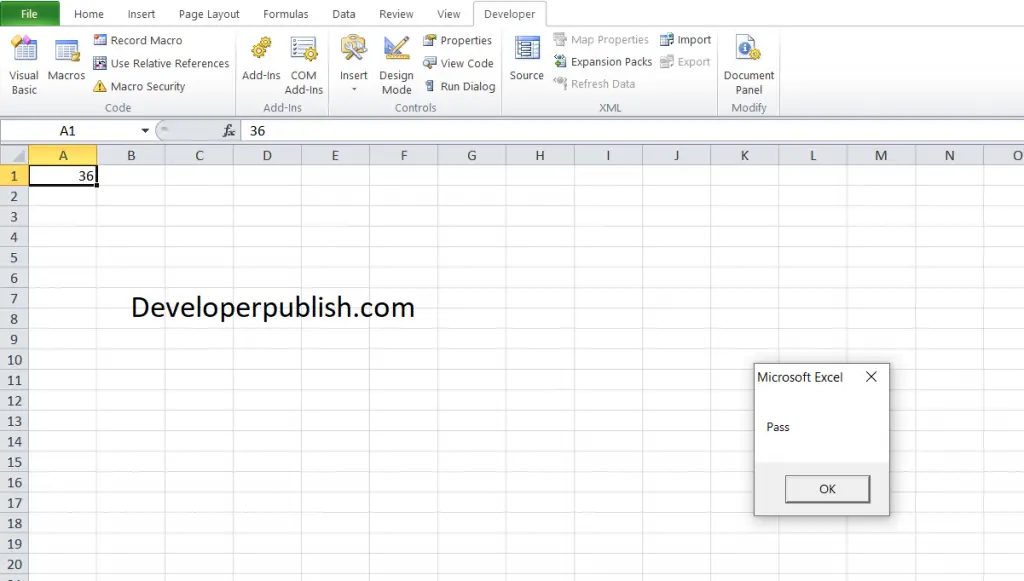
ElseIf
This VBA conditional statement is an If statement followed by one or more ElseIf statements that consists of boolean expressions and with a else statement at the ending.
Syntax
If(boolean_expression) Then   Statements ElseIf (boolean_expression) Then   Statements ElseIf (boolean_expression) Then   Statements Else   Statements End If
Sample program using elseif statement in VBA
Input
Private Sub if_demo_Click() Dim a As Integer Dim b As Integer a = 111 b = 123 If a > b Then MsgBox "a is Greater than b" ElseIf b > a Then MsgBox "b is Greater than a" Else MsgBox "a and b are EQUAL" End If End Sub
Output
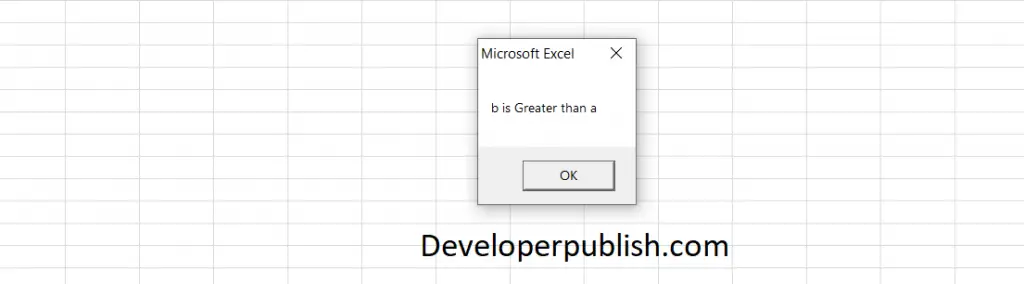
IF GO TO
With the help Goto we can go to any specified code of line or location in VBA.
Sample program using IF GOTO in VBA
Input
Sub GoTo_Example() Dim number As Integer number = 57 If number >= 100 Then GoTo Skip MsgBox "Number is lesser than 100" Skip: End Sub
Output
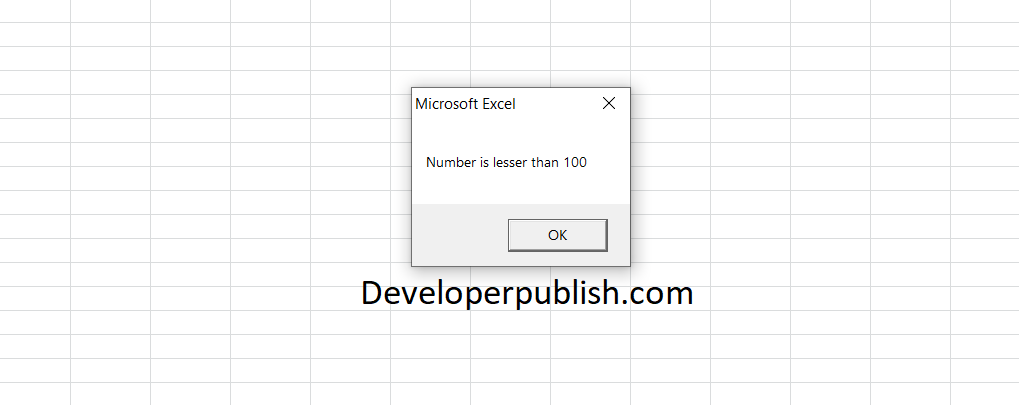
SELECT CASE
Select case in VBA is similar to If else statement. It helps in checking multiple conditions.
 Select Case Syntax
Select Case Test_Expression Case Value_1 Code Block when Test_Expression = Value_1 Case Value_2 Code Block when Test_Expression = Value_2 Case Value_3 Code Block when Test_Expression = Value_3 Case Else Code Block when none of the case conditions are met End Select
Sample program using select case statement in VBA
Input
Sub CheckNumber() Dim UserInput As Integer UserInput = InputBox("Please enter a number between 110 and 115") Select Case UserInput Case 111 MsgBox "You entered 111" Case 112 MsgBox "You entered 112" Case 113 MsgBox "You entered 113" Case 114 MsgBox "You entered 114" Case 115 MsgBox "You entered 115" End Select End Sub
Output
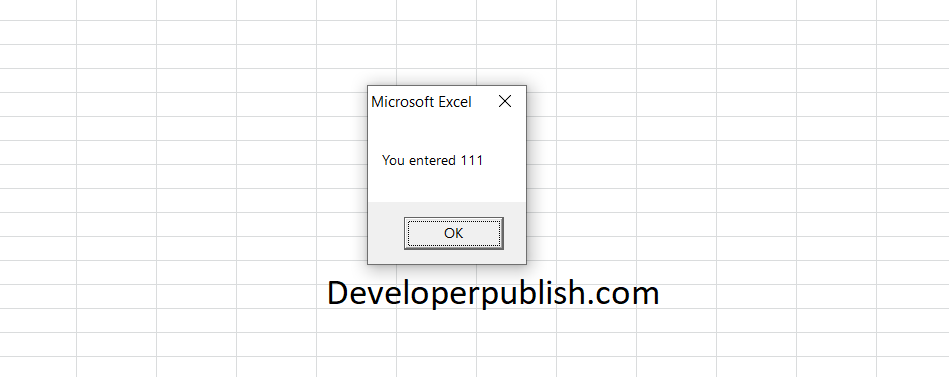