Do you want to get assembly version or assembly file version in your C# code?. This blog post exactly explains how you can do it with simple code.
When you check the Project properties your .NET Application , you would notice the following properties.
- AssemblyVersion
- AssemblyFileVersion
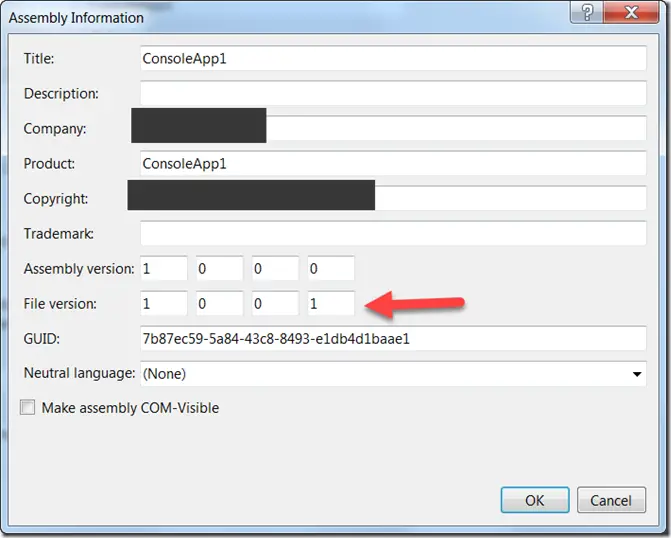
Its pretty easy to get the Assembly Version of the project using the Version property as shown below.
Assembly.GetEntryAssembly().GetName().Version;
but , how do one get the assembly file version number ?.
How to get the Assembly File Version in C# ?
When you right click on the assembly , you would be able to see the Assembly File version as shown below.
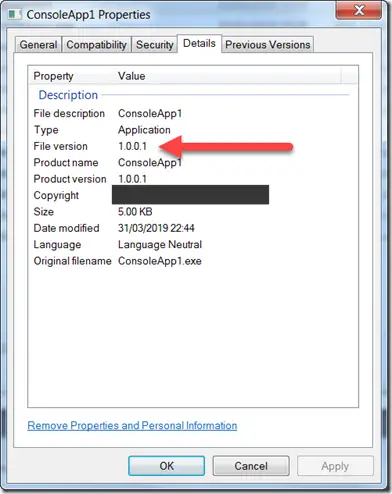
To get this property , you can use reflection and retreive it as shown below.
public static string GetAssemblyFileVersion() { System.Reflection.Assembly assembly = System.Reflection.Assembly.GetExecutingAssembly(); FileVersionInfo fileVersion = FileVersionInfo.GetVersionInfo(assembly.Location); return fileVersion.FileVersion; }
Full code snippet that is used for this demo is shown below.
using System; using System.Diagnostics; namespace ConsoleApp1 { class Program { static void Main(string[] args) { string version = GetAssemblyFileVersion(); Console.WriteLine(version); Console.ReadLine(); } public static string GetAssemblyFileVersion() { System.Reflection.Assembly assembly = System.Reflection.Assembly.GetExecutingAssembly(); FileVersionInfo fileVersion = FileVersionInfo.GetVersionInfo(assembly.Location); return fileVersion.FileVersion; } } }