Assume that you have a List of string and you wish you to get the items of the list into a comma separated string , you will be able to it easily do with the help of string.join method.
How to generate a comma seperated string from a list in C# ?
Assume that the list contains three items Senthil , Kumar , CredAbility as shown below.
IList<string> lst = new List<string>{"Senthil","Kumar","CredAbility"};
To get the comma seperated string of all the items in the list , use the string.Join as shown below.
string commaseperatedstring = string.Join(",", lst);
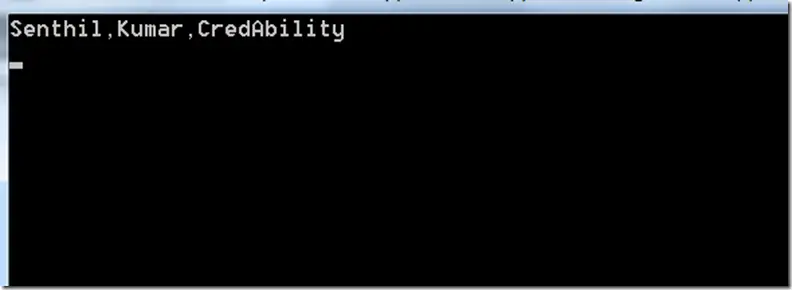
Here’s the full code snippet that is used in this example.
using System; using System.Collections.Generic; namespace ConsoleApp1 { class Program { static void Main(string[] args) { IList<string> lst = new List<string> { "Senthil", "Kumar", "CredAbility" }; string commaseperatedstring = string.Join(",", lst); Console.WriteLine(commaseperatedstring); Console.ReadLine(); } } }