In this example, we will create a C# program to find and print all the prime numbers between 1 and 100, demonstrating the language’s ability to handle prime number generation and basic loops.
Problem Statement
Write a C# program to find and print all the prime numbers between 1 and 100. A prime number is a positive integer greater than 1 that has no positive integer divisors other than 1 and itself.
C# Program to Print All the Prime Numbers between 1 to 100
using System; class Program { static void Main() { Console.WriteLine("Prime numbers between 1 and 100:"); for (int number = 2; number <= 100; number++) { if (IsPrime(number)) { Console.Write(number + " "); } } } static bool IsPrime(int num) { if (num <= 1) { return false; } for (int divisor = 2; divisor * divisor <= num; divisor++) { if (num % divisor == 0) { return false; } } return true; } }
Input / Output
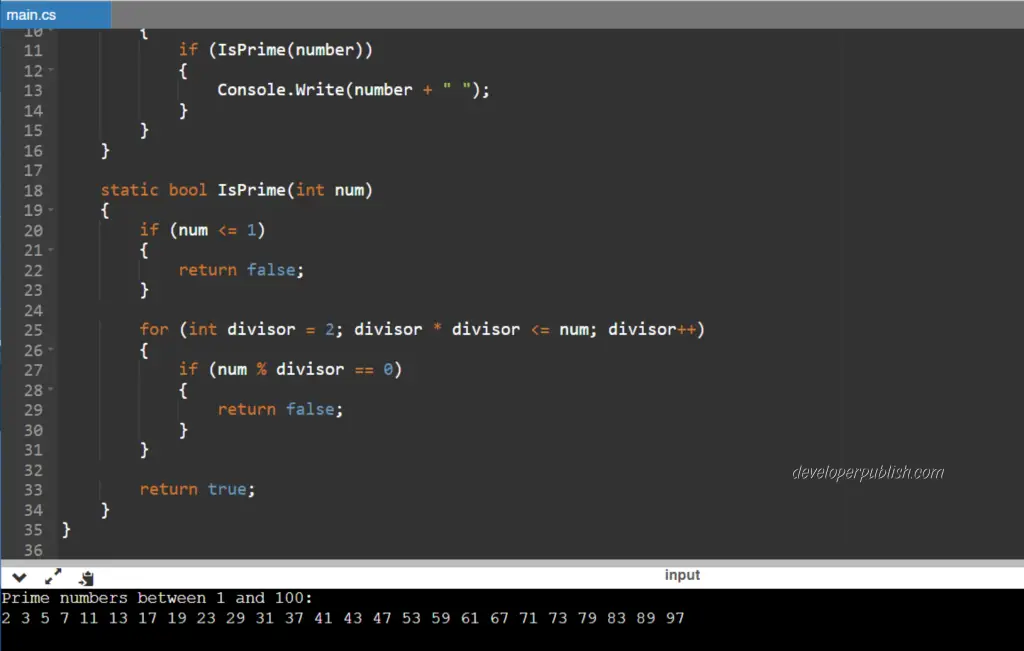