This C# Program demonstrates the basic arithmetic operations.
Problem Statement
Write a Program in C# demonstrating the basic arithmetic operations.
You can choose from the following operations:
- Addition (+)
- Subtraction (-)
- Multiplication (*)
- Division (/)”);
C# Program to Perform All Arithmetic Operations
using System; class Program { static void Main() { double num1, num2; char operatorSymbol; Console.WriteLine("Arithmetic Operations Program"); Console.WriteLine("============================="); Console.Write("Enter the first number: "); num1 = GetValidNumber(); Console.Write("Enter the second number: "); num2 = GetValidNumber(); Console.Write("Enter the operator (+, -, *, /): "); operatorSymbol = GetValidOperator(); double result = 0.0; switch (operatorSymbol) { case '+': result = num1 + num2; break; case '-': result = num1 - num2; break; case '*': result = num1 * num2; break; case '/': if (num2 == 0) { Console.WriteLine("Error: Division by zero is not allowed."); return; } result = num1 / num2; break; default: Console.WriteLine("Invalid operator entered."); return; } Console.WriteLine($"Result: {num1} {operatorSymbol} {num2} = {result}"); } static double GetValidNumber() { double number; while (!double.TryParse(Console.ReadLine(), out number)) { Console.Write("Invalid input. Please enter a valid number: "); } return number; } static char GetValidOperator() { char[] validOperators = { '+', '-', '*', '/' }; char operatorSymbol; while (!char.TryParse(Console.ReadLine(), out operatorSymbol) || Array.IndexOf(validOperators, operatorSymbol) == -1) { Console.Write("Invalid input. Please enter a valid operator (+, -, *, /): "); } return operatorSymbol; } }
How it works
- The program starts with an introduction and displays a menu of available arithmetic operations.
- The user is prompted to enter two numbers and select an operation by entering the corresponding number (1-4) for addition, subtraction, multiplication, or division.
- The program then performs the selected operation and displays the result.
- If the user selects division and the second number is 0, the program prevents division by zero.
- Finally, a program completion message is displayed.
Input / Output
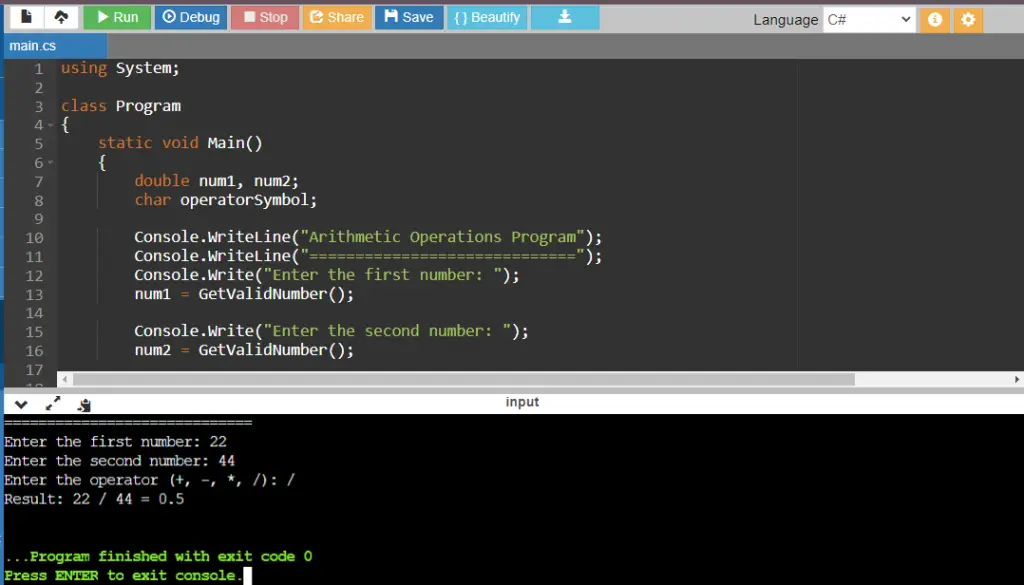