This C# program is designed to determine the minimum and maximum values within an array of integers. Finding the minimum and maximum values in a dataset is a common task in programming and data analysis. These values can provide essential insights into the data’s range and characteristics.
Problem statement
You are given an array of integers. Write a C# program that finds the minimum and maximum numbers in the array.
C# Program to Find Minimum and Maximum of Numbers in an Array
using System; class Program { static void FindMinMax(int[] arr, out int min, out int max) { if (arr.Length == 0) { throw new ArgumentException("The array cannot be empty"); } min = max = arr[0]; foreach (int num in arr) { if (num < min) { min = num; } if (num > max) { max = num; } } } static void Main() { int[] numbers = { 4, 2, 9, 7, 5, 1, 8, 3, 6 }; int min, max; FindMinMax(numbers, out min, out max); Console.WriteLine("Minimum number: " + min); Console.WriteLine("Maximum number: " + max); } }
How it works
The program is written in C# and is designed to find the minimum and maximum numbers in an array of integers. Here’s how it works:
- Method Definition (
FindMinMax
):- The program defines a method named
FindMinMax
that takes three parameters: an array of integers (arr
), and twoout
parameters (min
andmax
) which will be used to store the minimum and maximum values. - If the array is empty, it throws an
ArgumentException
with the message “The array cannot be empty”.
- The program defines a method named
- Variable Initialization:
- Within
FindMinMax
,min
andmax
are initially set to the first element of the array (arr[0]
). This provides a starting point for comparison.
- Within
- Iterating Through the Array:
- A
foreach
loop is used to iterate through each element (num
) in the arrayarr
.
- A
- Comparing and Updating:
- For each element, the program checks if
num
is less thanmin
. If so, it updatesmin
to be equal.
- For each element, the program checks if
Input/Output
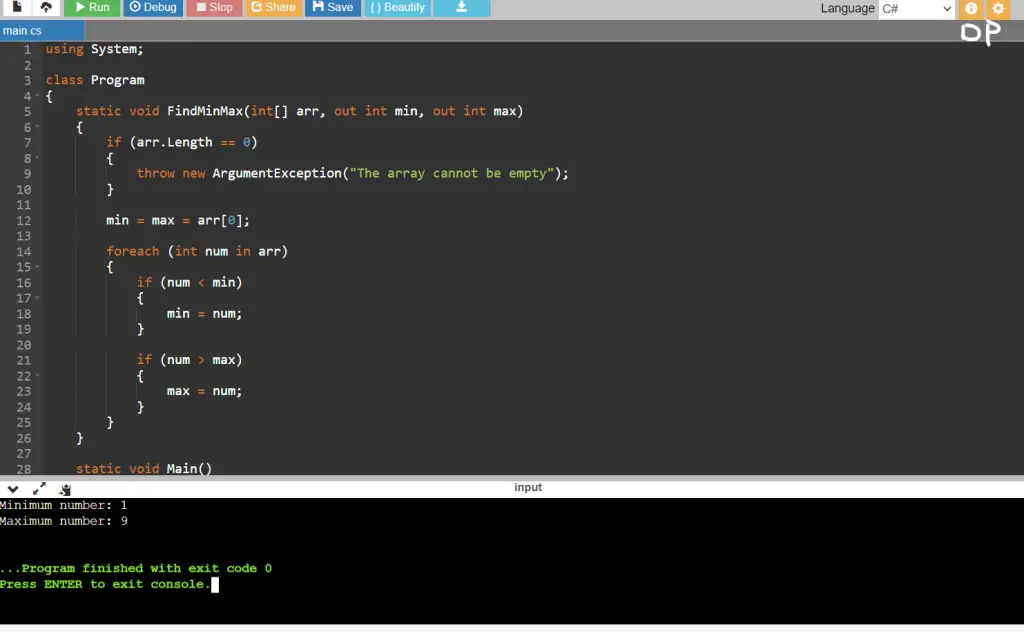