This C program finds the Greatest Common Divisor (GCD) and the Least Common Multiple (LCM) of two given numbers using the Euclidean Algorithm.
Problem statement
Write a C program that takes two numbers as input from the user and uses the Euclidean algorithm to find their greatest common divisor (GCD) and least common multiple (LCM). The program should then output the calculated GCD and LCM.
Your program should follow these steps:
- Prompt the user to enter two numbers.
- Read the two numbers from the user.
- Implement a function named
gcd
that takes two integer parameters and returns their GCD using the Euclidean algorithm. - Implement a function named
lcm
that takes two integer parameters and returns their LCM using the GCD. - In the
main
function, call thegcd
andlcm
functions with the user-provided numbers as arguments. - Output the calculated GCD and LCM to the console
Note:
Ensure that the program can handle positive integer inputs
C Program to Find GCD and LCM of Two Numbers using Euclidean Algorithm
#include <stdio.h> // Function to calculate the GCD of two numbers using the Euclidean algorithm int gcd(int a, int b) { if (b == 0) { return a; } else { return gcd(b, a % b); } } // Function to calculate the LCM of two numbers int lcm(int a, int b) { return (a * b) / gcd(a, b); } int main() { int num1, num2; printf("Enter two numbers: "); scanf("%d %d", &num1, &num2); int gcdResult = gcd(num1, num2); int lcmResult = lcm(num1, num2); printf("GCD: %d\n", gcdResult); printf("LCM: %d\n", lcmResult); return 0; }
How it works
- The program starts by prompting the user to enter two numbers.
- The
scanf
function is used to read the two numbers from the user and store them in variablesnum1
andnum2
. - The program then calls the
gcd
function withnum1
andnum2
as arguments. Thegcd
function uses the Euclidean algorithm to recursively calculate the GCD of the two numbers.- If
b
(the second number) is 0, it means thata
(the first number) is the GCD, and the function returnsa
. - Otherwise, the function calls itself with
b
as the first argument anda % b
as the second argument.
- If
- The program assigns the returned GCD value from the
gcd
function to the variablegcdResult
. - The program then calls the
lcm
function withnum1
andnum2
as arguments to calculate the LCM.- The
lcm
function uses the formula(a * b) / gcd(a, b)
to calculate the LCM.
- The
- The program assigns the returned LCM value from the
lcm
function to the variablelcmResult
. - Finally, the program prints the calculated GCD and LCM values to the console using
printf
statements.
By utilizing the Euclidean algorithm to find the GCD and the LCM formula, the program efficiently calculates and displays the GCD and LCM of the given input numbers.
Input / Output
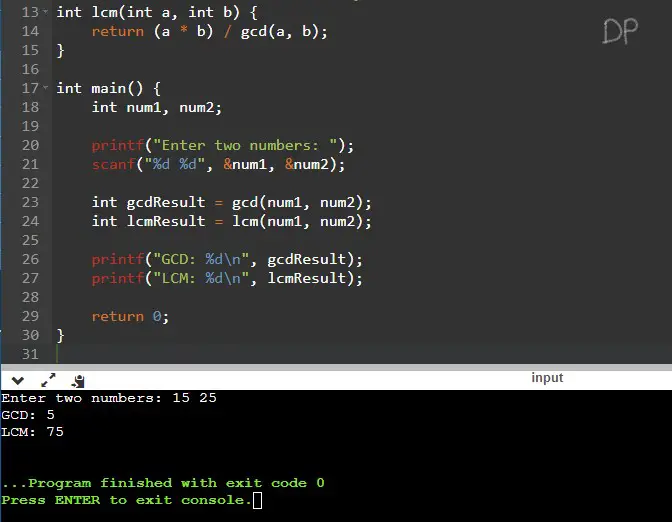