C# is a versatile programming language widely used in various applications. In this example, we will explore a straightforward C# program to determine whether a given number is positive, demonstrating the language’s ability to perform basic conditional checks.
Problem Statement
Write a C# program to check whether a given number is positive or not. The program should take an input number and determine if it is greater than zero. If it is, the number is considered positive; otherwise, it is not.
C# Program to Check Whether a Number is Positive or Not
using System; class Program { static void Main() { Console.Write("Enter a number: "); double number = Convert.ToDouble(Console.ReadLine()); if (IsPositive(number)) { Console.WriteLine(number + " is a positive number."); } else { Console.WriteLine(number + " is not a positive number."); } } static bool IsPositive(double num) { return num > 0; } }
Input / Output
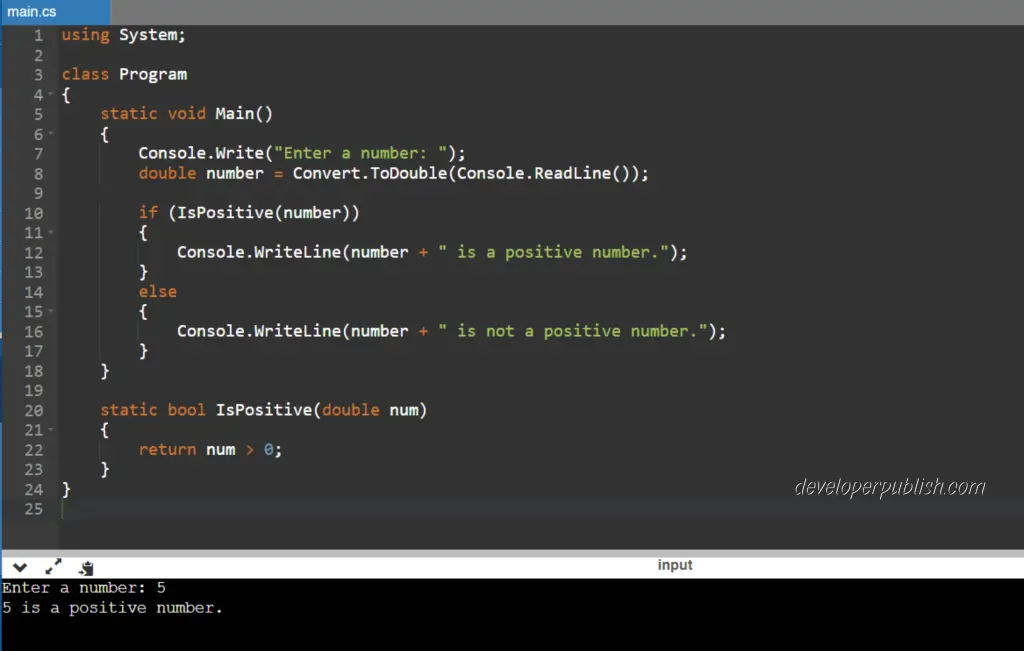