C# Compiler Error
CS0545 – ‘function’ : cannot override because ‘property’ does not have an overridable get accessor
Reason for the Error
You will get this error in your C# code when you attempt to override a property that does not have an get accessor.
For example, let’s compile the below C# program
using System; namespace DeveloperPublishConsoleCore { public class Employee { public virtual int Id { set { } } } public class Contract : Employee { public override int Id { get { return 0; } set { } } } internal class Program { static void Main(string[] args) { Console.WriteLine("DeveloperPublish Hello World!"); } } }
You will receive the error code CS0545 because the class Employee contains a Virtual property “Id” without an get accessor. You are attempting to override it in the Contract class with the getter.
Error CS0545 ‘Contract.Id.get’: cannot override because ‘Employee.Id’ does not have an overridable get accessor DeveloperPublishConsoleCore C:\Users\senth\source\repos\DeveloperPublishConsoleCore\DeveloperPublishConsoleCore\Program.cs 17 Active
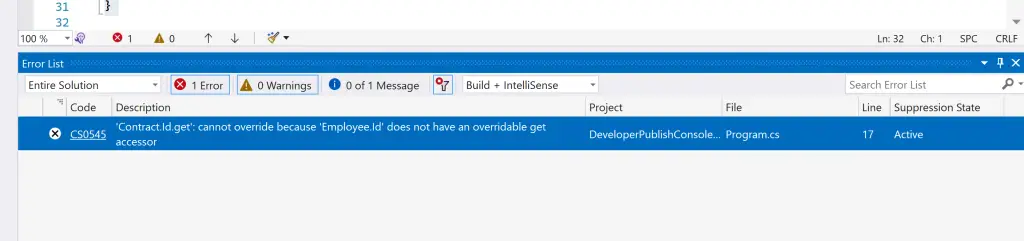
Solution
To fix this error in your C# program, you can add the get accessor in the base class or remove the get accessor from the derived or child class. Alternatively, you can use the new keyword to hide the base class property.