C# Compiler Error
CS0544 – ‘property override’: cannot override because ‘non-property’ is not a property
Reason for the Error
You will get this error in your C# code when you attempt to override a non-property member (Eg : field) as property.
For example, let’s compile the below C# program
using System; namespace DeveloperPublishConsoleCore { public class Employee { public int id; } public class Contract : Employee { // Results in the Error Code CS0544 public override int id { get { return 0; } } } internal class Program { static void Main(string[] args) { Console.WriteLine("DeveloperPublish Hello World!"); } } }
You will receive the error code CS0544 because you have a field id in the Employee class and you are attempting to override it as property in the Contract class.
Error CS0544 ‘Contract.id’: cannot override because ‘Employee.id’ is not a property DeveloperPublishConsoleCore C:\Users\senth\source\repos\DeveloperPublishConsoleCore\DeveloperPublishConsoleCore\Program.cs 13 Active
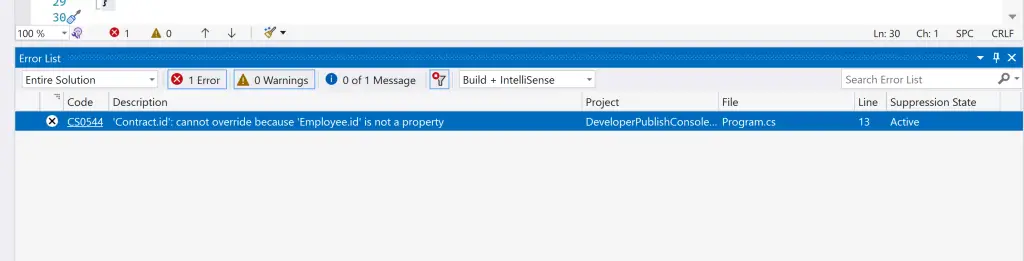
Solution
C# compiler does not allow you to override a ‘non-property’ as property. To fix this error in your C# program, ensure that you override the right type.