C# Compiler Error
CS0248 – Cannot create an array with a negative size
Reason for the Error
You will receive this error in C# when you are trying to create an array with a negative number being specified to it.
For example, lets try to compile the below code snippet.
namespace DeveloperPubNamespace { class Program { public static void Main() { unsafe { int[] array1 = new int[-15]; } } } }
You will receive the error code CS0248 in your C# program because you are trying to create an array of integer by specifying the size of the array to be -15. This is invalid in the context of array and hence C# identifies this as error.
Error CS0248 Cannot create an array with a negative size DeveloperPublish C:\Users\SenthilBalu\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 10 Active
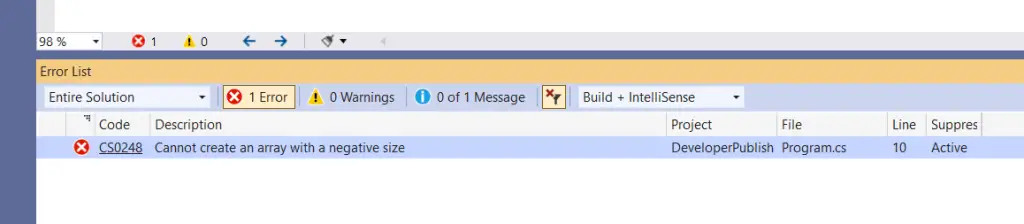
Solution
The array size in C# should always be a positive number. To fix the error code CS0248, ensure that you always pass a positive number when creating an array.
namespace DeveloperPubNamespace { class Program { public static void Main() { { int[] array1 = new int[15]; } } } }