C# Compiler Error
CS0246 – The type or namespace name ‘type/namespace’ could not be found (are you missing a using directive or an assembly reference?)
Reason for the Error
You will most likely receive this error when the C# compiler has not found the type or the namespace that is used in your C# program.
There are plenty of reasons why you will have this error code from C#. These include
- You have missed adding reference to the assembly that contains the type.
- You have missed adding the required using directive.
- You could have misspell the name of the type or namespace.
- You would have referenced an assembly that was built against a higher .NET framework version than the target version of the current program.
For example, try compiling the below code snippet.
using System; namespace DeveloperPubNamespace { class Program { public static void Main() { var lstData = new List<int>(); } } }
This program will result with the C# error code CS0246 because you are using the type List but is not fully qualified or the using directive is not applied in the program for the namespace where the List exists.
Error CS0246 The type or namespace name ‘List<>’ could not be found (are you missing a using directive or an assembly reference?) DeveloperPublish C:\Users\SenthilBalu\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 9 Active
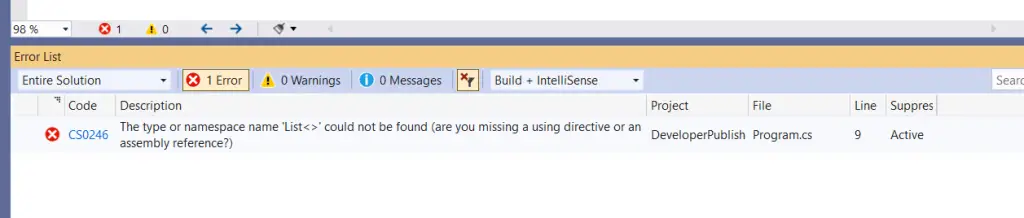
Solution
You can fix this error in C# by ensuring that you add the right type or namespace in your program. Ensure that you have used the using directive to include the namespace or atleast using the fully qualified name for the class as shown below.
namespace DeveloperPubNamespace { class Program { public static void Main() { var lstData = new System.Collections.Generic.List<int>(); } } }