C# Compiler Error
CS0216 – The operator ‘operator’ requires a matching operator ‘missing_operator’ to also be defined
Reason for the Error
You will receive this error in C# when you are working with the user defined operators and missed defining the required operator.
For example, when you define a user defined operator for true operator, you’ll need to define the false operator as well. The same applies for == and != operators.
For example, try to compile the below code snippet.
namespace DeveloperPubNamespace { class Program { public static bool operator true(Program obj) { return true; } static void Main() { } } }
This program will result with the error code CS0216 because we have defined the user defined true operator but missed the false operator.
Error CS0216 The operator ‘Program.operator true(Program)’ requires a matching operator ‘false’ to also be defined ConsoleApp3 C:\Users\SenthilBalu\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 5 Active
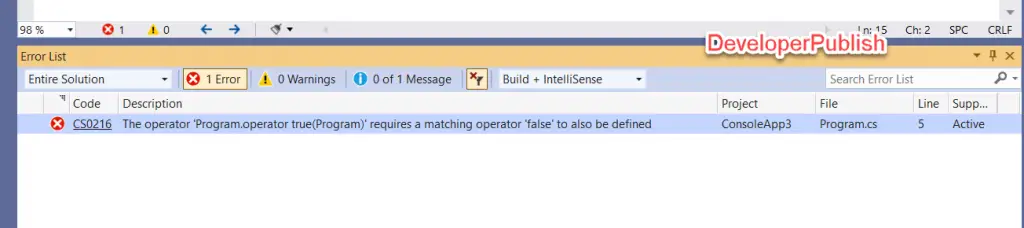
Solution
When you are working with the user defined operators in C#, you’ll need to ensure that you define the required operators. You can fix the error in your C# program by defining the missing operator (Eg : false in the above program) as demonstrated in the below code snippet.
namespace DeveloperPubNamespace { class Program { public static bool operator true(Program obj) { return true; } public static bool operator false(Program obj) { return false; } static void Main() { } } }