This post will explain how you can check if a string is null or string contains only whitespace orin C#.
How to check if string is null or contains only whitespaces in C# ?
In the the previous Versions of the C# ,To check if the string is null or empty , we do it something similar to the example stated below.
if (input == null || (input == "") { Console.WriteLine("The string is Null or Empty"); }
The method string.IsNullOrEmpty in earlier versions of C# , enables to check for the string if it is null or empty .
Eg : if (string.IsNullOrEmpty(input )) { Console.WriteLine("The string is Null or Empty"); }
The code looked much cleaner with IsNullOrEmpty 🙂
However the method doesnot work if the string contains only whitespaces. Whitespaces refers to the characters like space, line break, tab and empty string etc.
Eg :
if (string.IsNullOrEmpty(' '.ToString())) { MessageBox.Show("emptyorNull"); }
The above code will return false .We have to use the Trim() method to get it to work.
if (string.IsNullOrEmpty(' '.ToString().Trim())) { MessageBox.Show("emptyorNull"); }
C# 4.0 makes it easy with the new method String.IsNullOrWhiteSpace .This method finds out if the specified string contains null, empty, or consists only of white-space characters and returns the true/false accordingly .
string input = new String(' ', 5); if (String.IsNullOrWhiteSpace(input)) { MessageBox.Show("String is Empty or Null or has only White Spaces"); }
The above example will return true.
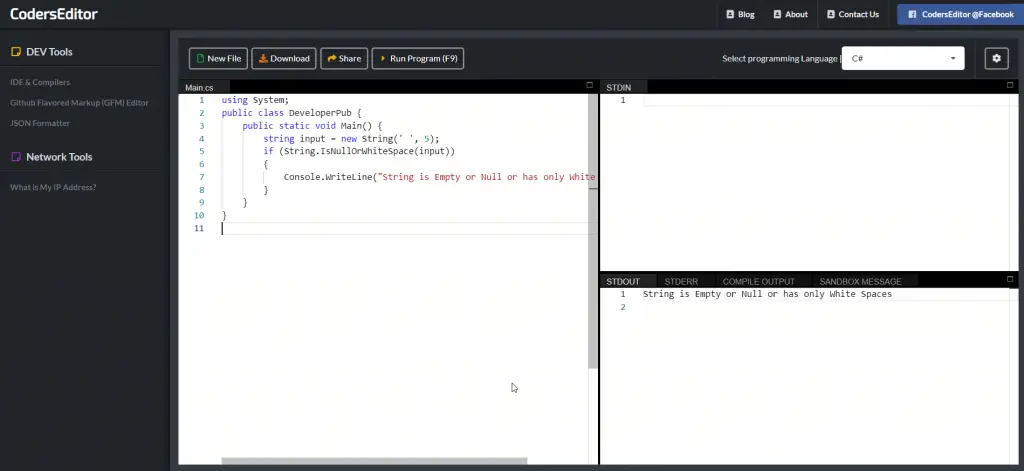
2 Comments
very handy methods
Good Work