This Python program reverses a given string without using recursion. It uses a simple iterative approach to reverse the string.
In this block, we present a Python program to reverse a given string without using recursion. This alternative method provides an efficient solution for reversing strings, avoiding potential performance issues and stack overflow errors.
Problem statement
Write a Python program that reverses a given string without using recursion. The program should take a string as input and output the reversed version of the string.
Python Program to Reverse a String Without using Recursion
def reverse_string(input_str): reversed_str = "" for char in input_str: reversed_str = char + reversed_str return reversed_str # Test the function user_input = input("Enter a string: ") reversed_input = reverse_string(user_input) print("Reversed String:", reversed_input)
Input/Output
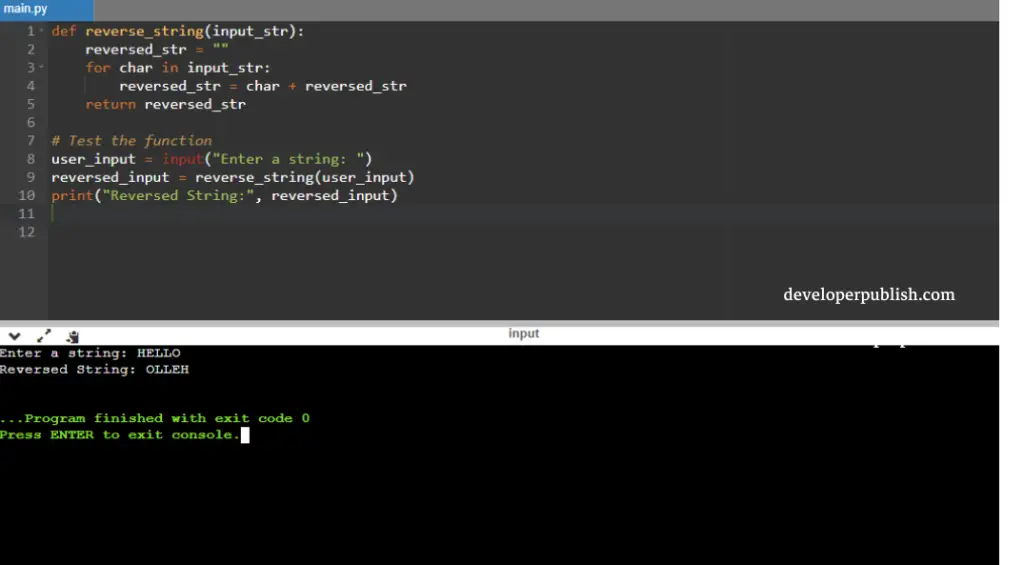