This program demonstrates how to remove a key from a dictionary in Python.
Problem Statement:
Given a dictionary, remove a specific key and its corresponding value from the dictionary in python.
Python Program to Remove a Key from a Dictionary
def remove_key(dictionary, key_to_remove): if key_to_remove in dictionary: del dictionary[key_to_remove] print(f"Key '{key_to_remove}' and its value removed from the dictionary.") else: print(f"Key '{key_to_remove}' not found in the dictionary.") # Example dictionary my_dict = {'a': 1, 'b': 2, 'c': 3} # Key to remove key_to_remove = 'b' remove_key(my_dict, key_to_remove) print("Updated Dictionary:", my_dict)
How It Works:
- The program defines a function
remove_key
which takes a dictionary and a key as arguments. - Inside the function, it checks if the given key exists in the dictionary using the
in
operator. - If the key exists, it uses the
del
statement to remove the key-value pair from the dictionary. - If the key is not found, it prints a message indicating that the key was not found.
- The program then creates an example dictionary
my_dict
and specifies a keykey_to_remove
that needs to be removed. - The
remove_key
function is called with themy_dict
andkey_to_remove
as arguments. - Finally, the updated dictionary is printed.
Input/Output:
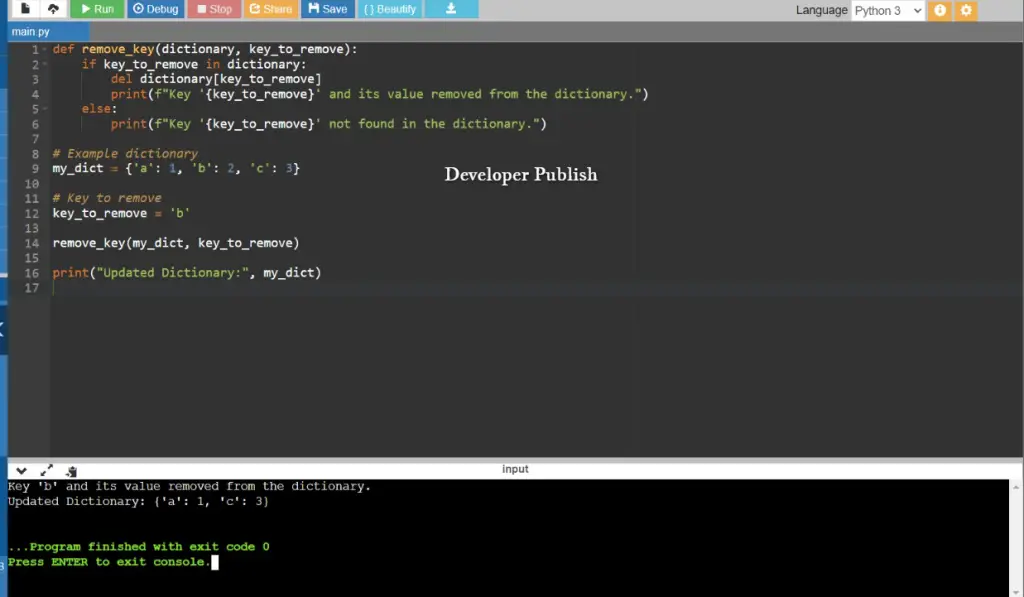