Python dictionaries provide a convenient way to map keys to values. This program demonstrates how to create a Python program that maps two lists into a dictionary, where elements from one list act as keys and elements from another list act as values.
Problem Statement
Write a Python program that takes two lists as input and maps them into a dictionary, where elements from the first list become keys and elements from the second list become values.
Python Program to Map Two Lists into a Dictionary
def map_lists_to_dictionary(keys, values): result_dict = {} for i in range(len(keys)): result_dict[keys[i]] = values[i] return result_dict # Input keys_list = input("Enter the keys list (comma-separated values): ").split(',') values_list = input("Enter the values list (comma-separated values): ").split(',') # Mapping lists to dictionary mapped_dict = map_lists_to_dictionary(keys_list, values_list) # Output print("Mapped dictionary:", mapped_dict)
Input / Output
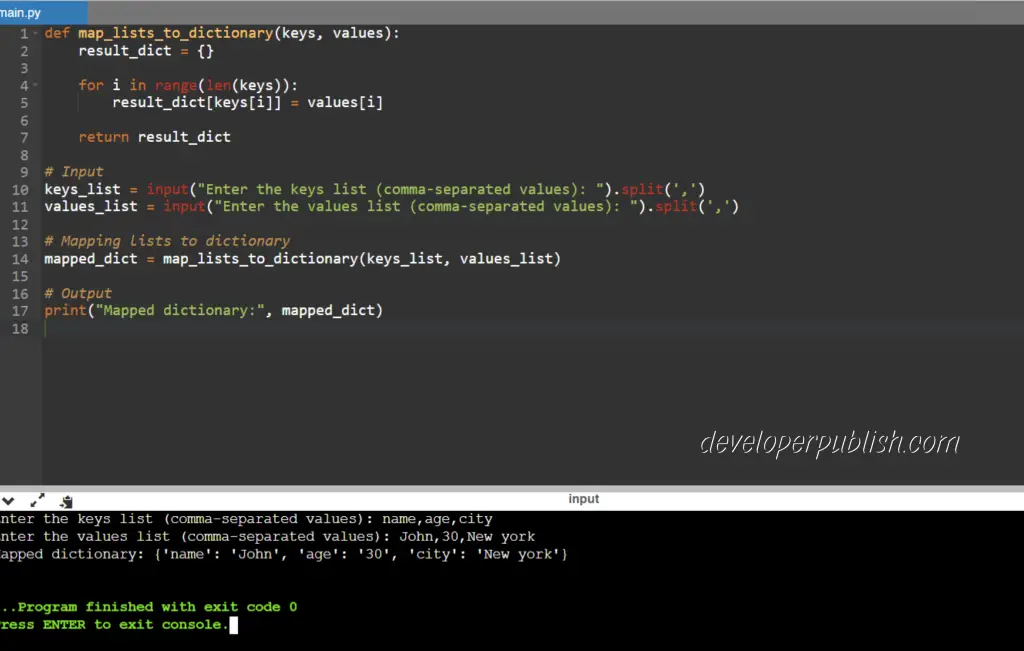