A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward. This program illustrates how to create a Python program to determine whether a given string is a palindrome or not.
Problem Statement
Write a Python program that takes a string as input and checks whether it is a palindrome or not.
Python Program to Check if a Given String is Palindrome
def is_palindrome(string): string = string.lower() # Convert to lowercase for case-insensitive comparison reversed_string = string[::-1] return string == reversed_string # Input input_string = input("Enter a string: ") # Checking for palindrome if is_palindrome(input_string): print("The given string is a palindrome.") else: print("The given string is not a palindrome.")
Input / Output
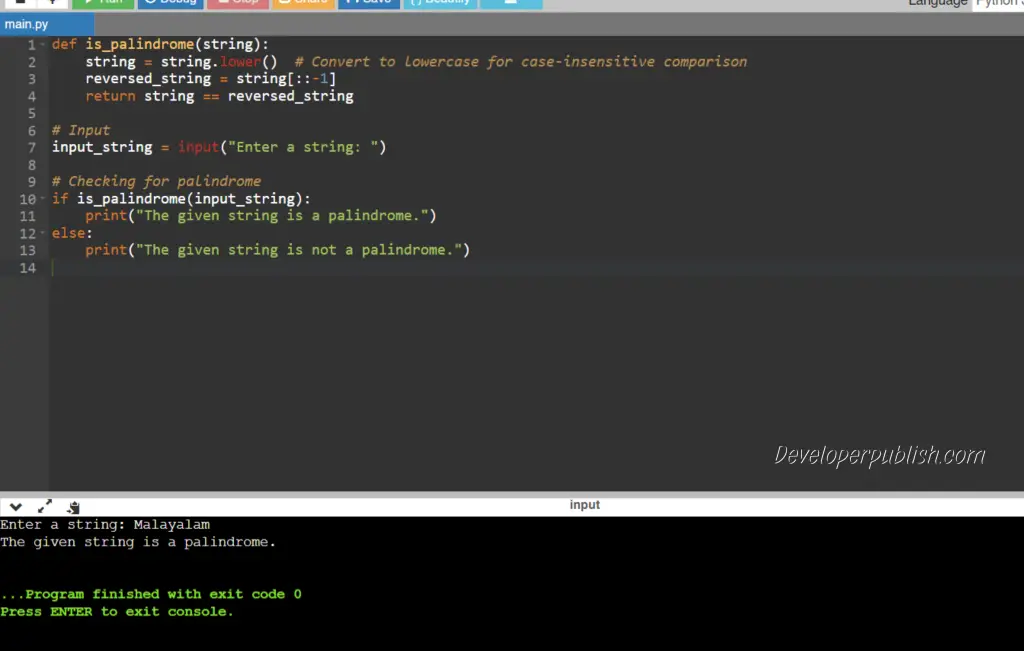