In this C# tips & tricks post , we will look at a simple usage of LINQ in C# to split list into multiple list with the extension method.
How to Split list into multiple list in C# ?
Assume that you have a ListSize which you will need to split the list. For example , if you have a list with 4 elements and you wish to convert them in to 4 different lists. Here’s a code snippet demonstrating how you can do that.
public static class TestExtensionMethod { public static IEnumerable<IEnumerable<T>> Split<T>(this IEnumerable<T> sourceList, int ListSize) { while (sourceList.Any()) { yield return sourceList.Take(ListSize); sourceList = sourceList.Skip(ListSize); } } }
Here’s the full code snippet.
using System; using System.Collections.Generic; using System.Linq; namespace DeveloperPublish { internal class Program { private static void Main(string[] args) { List<string> Names = new List<string> { "Senthil Kumar" , "Ashwini Sambandan" , "Norton Stanley" , "Santhosh" }; var result = Names.Split(1).ToList(); Console.WriteLine(result[0]); Console.ReadLine(); } } public static class TestExtensionMethod { public static IEnumerable<IEnumerable<T>> Split<T>(this IEnumerable<T> sourceList, int ListSize) { while (sourceList.Any()) { yield return sourceList.Take(ListSize); sourceList = sourceList.Skip(ListSize); } } } }
Output
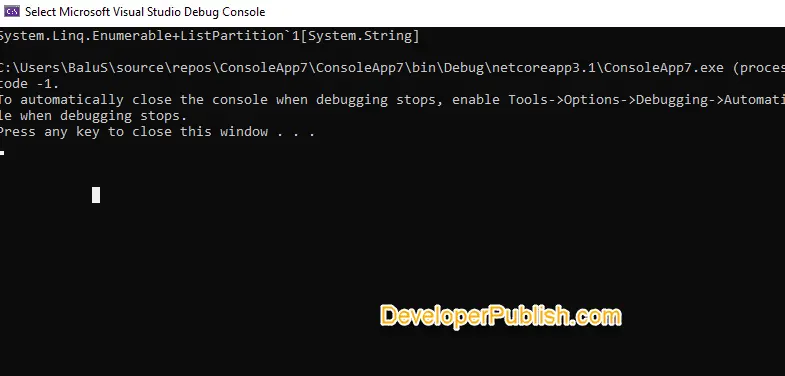