You can easily convert the C# object to JSON string by using the Newtonsoft.Json NuGet package which is one of the popular serializer for the .NET Developers.
How to convert C# object to JSON string in C# ?
1. Ensure that the Newtonsoft.Json NuGet package is installed in the project.
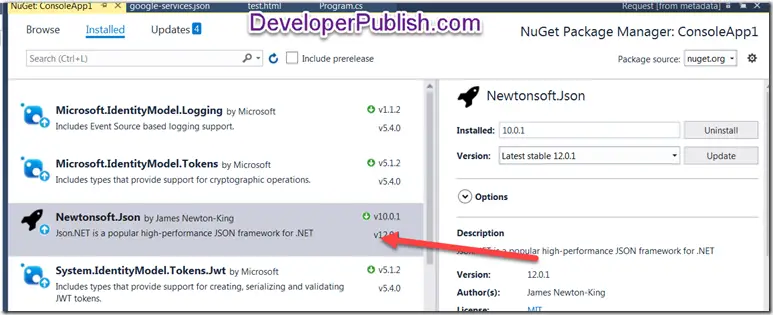
2. Assuming that you have a C# class with the following properties and you want them to be serialized.
public class Employee { public string FirstName { get; set; } public string LastName { get; set; } public DateTime JoinedDate { get; set; } }
3. You can serialize or convert the C# object to Json string with the SerializeObject method
Employee emp = new Employee(); emp.FirstName = "Senthil"; emp.LastName = "Balu"; emp.JoinedDate = DateTime.Now; var jsonString = Newtonsoft.Json.JsonConvert.SerializeObject(emp);
Here’s the full code snippet demonstrating how to serialize the C# class and get the Json string.
using System; using System.Collections.Generic; namespace ConsoleApp1 { public class Employee { public string FirstName { get; set; } public string LastName { get; set; } public DateTime JoinedDate { get; set; } } class Program { static void Main(string[] args) { Employee emp = new Employee(); emp.FirstName = "Senthil"; emp.LastName = "Balu"; emp.JoinedDate = DateTime.Now; var jsonString = Newtonsoft.Json.JsonConvert.SerializeObject(emp); Console.WriteLine(jsonString); Console.ReadLine(); } } }
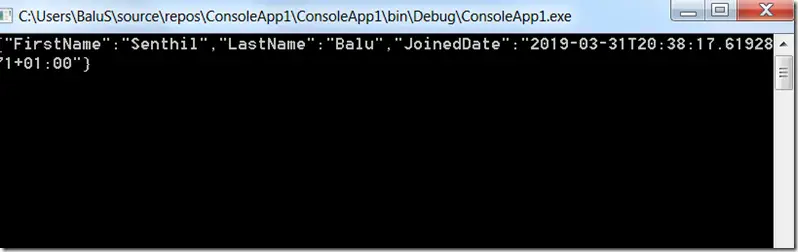